Flows and Views
The OWUIFlows
and OWUIViews
protocols provide two approaches to add the Conversation module and the general UI to your app: Flows and Views.
Either approach enables you to incorporate elements, such as PreConversation, Conversation, and Comment Creation experiences.
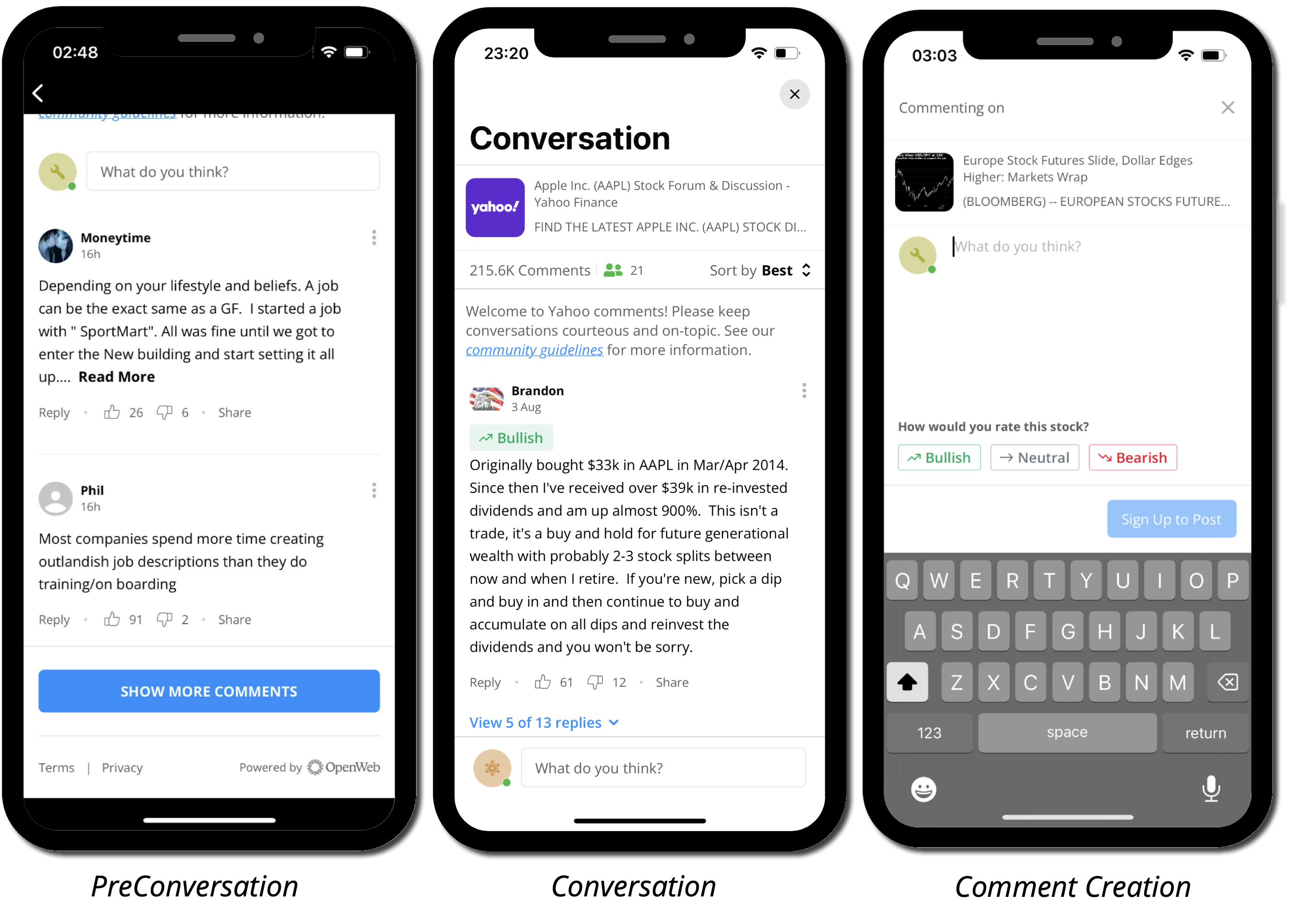
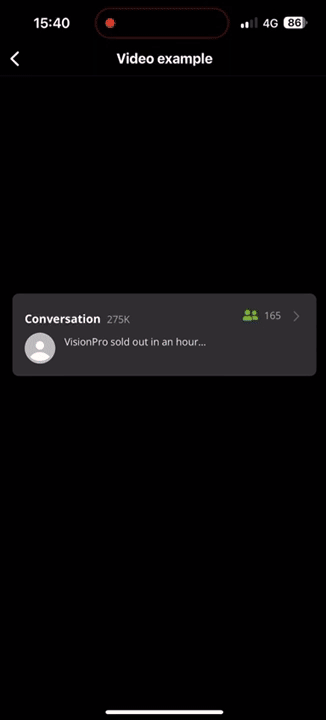
Conversation below video
public protocol OWUIFlows {
func preConversation(postId: OWPostId,
article: OWArticleProtocol,
presentationalMode: OWPresentationalMode,
additionalSettings: OWAdditionalSettingsProtocol,
callbacks: OWFlowActionsCallbacks?,
completion: @escaping OWViewCompletion)
func conversation(postId: OWPostId,
article: OWArticleProtocol,
presentationalMode: OWPresentationalMode,
additionalSettings: OWAdditionalSettingsProtocol,
callbacks: OWFlowActionsCallbacks?,
completion: @escaping OWDefaultCompletion)
func commentCreation(postId: OWPostId,
article: OWArticleProtocol,
presentationalMode: OWPresentationalMode,
additionalSettings: OWAdditionalSettingsProtocol,
callbacks: OWFlowActionsCallbacks?,
completion: @escaping OWDefaultCompletion)
func commentThread(postId: OWPostId,
article: OWArticleProtocol,
commentId: OWCommentId,
presentationalMode: OWPresentationalMode,
additionalSettings: OWAdditionalSettingsProtocol,
callbacks: OWFlowActionsCallbacks?,
completion: @escaping OWDefaultCompletion)
}
public protocol OWUIFlows {
@MainActor
func preConversation(postId: OWPostId,
article: OWArticleProtocol,
presentationalMode: OWPresentationalMode,
additionalSettings: OWAdditionalSettingsProtocol,
callbacks: OWFlowActionsCallbacks?) async throws -> UIView
@MainActor
func conversation(postId: OWPostId,
article: OWArticleProtocol,
presentationalMode: OWPresentationalMode,
additionalSettings: OWAdditionalSettingsProtocol,
callbacks: OWFlowActionsCallbacks?) async throws
@MainActor
func commentCreation(postId: OWPostId,
article: OWArticleProtocol,
presentationalMode: OWPresentationalMode,
additionalSettings: OWAdditionalSettingsProtocol,
callbacks: OWFlowActionsCallbacks?) async throws
@MainActor
func commentThread(postId: OWPostId,
article: OWArticleProtocol,
commentId: OWCommentId,
presentationalMode: OWPresentationalMode,
additionalSettings: OWAdditionalSettingsProtocol,
callbacks: OWFlowActionsCallbacks?) async throws
}
public protocol OWUIViews {
func preConversation(postId: OWPostId,
article: OWArticleProtocol,
additionalSettings: OWAdditionalSettingsProtocol,
callbacks: OWViewActionsCallbacks?,
completion: @escaping OWViewCompletion)
func conversation(postId: OWPostId,
article: OWArticleProtocol,
additionalSettings: OWAdditionalSettingsProtocol,
callbacks: OWViewActionsCallbacks?,
completion: @escaping OWViewCompletion)
func commentCreation(postId: OWPostId,
article: OWArticleProtocol,
commentCreationType: OWCommentCreationType,
additionalSettings: OWAdditionalSettingsProtocol,
callbacks: OWViewActionsCallbacks?,
completion: @escaping OWViewCompletion)
func commentThread(postId: OWPostId,
article: OWArticleProtocol,
commentId: OWCommentId,
additionalSettings: OWAdditionalSettingsProtocol,
callbacks: OWViewActionsCallbacks?,
completion: @escaping OWViewCompletion)
func reportReason(postId: OWPostId,
commentId: OWCommentId,
parentId: OWCommentId,
additionalSettings: OWAdditionalSettingsProtocol,
callbacks: OWViewActionsCallbacks?,
completion: @escaping OWViewCompletion)
}
public protocol OWUIViews {
@MainActor
func preConversation(postId: OWPostId,
article: OWArticleProtocol,
additionalSettings: OWAdditionalSettingsProtocol,
callbacks: OWViewActionsCallbacks?) async throws -> UIView
@MainActor
func conversation(postId: OWPostId,
article: OWArticleProtocol,
additionalSettings: OWAdditionalSettingsProtocol,
callbacks: OWViewActionsCallbacks?) async throws -> UIView
@MainActor
func commentCreation(postId: OWPostId,
article: OWArticleProtocol,
commentCreationType: OWCommentCreationType,
additionalSettings: OWAdditionalSettingsProtocol,
callbacks: OWViewActionsCallbacks?) async throws -> UIView
@MainActor
func commentThread(postId: OWPostId,
article: OWArticleProtocol,
commentId: OWCommentId,
additionalSettings: OWAdditionalSettingsProtocol,
callbacks: OWViewActionsCallbacks?) async throws -> UIView
@MainActor
func reportReason(postId: OWPostId,
commentId: OWCommentId,
parentId: OWCommentId,
additionalSettings: OWAdditionalSettingsProtocol,
callbacks: OWViewActionsCallbacks?) async throws -> UIView
}
Each implementation approach is explained in the Approaches section.
Approaches
Flows
This approach relies upon OpenWeb's default UI.
When implementing a Conversation with the Flows approach, you should configure one of the three flows in the following table.
View | Description |
---|---|
PreConversation | Show a preview of comments at the end of an article to encourage engagement with a Conversation
This is an entry point if to the full-page Conversation experience. |
Conversation | Show a full-page Conversation experience that initiates when the user triggers a call-to-action (CTA) button |
Comment Creation | Navigate directly to a page in which the end user can create a new comment |
Comment Thread | Show a comment thread, starting from the root comment (commentId ) |
Flow Recipes
Use the following recipes to configure one of the flows.
iOS SDK PreConversation Flow
Use async-await
async-await
Use an initializer or builder
iOS SDK Conversation Flow
Use async-await
async-await
Use an initializer or builder
iOS SDK Comment Creation Flow
Use async-await
async-await
Use an initializer or builder
iOS SDK Comment Thread Flow
Use async-await
async-await
Use an initializer or builder
Views
Implementing a Conversation module with the Views approach, gives you the ability to control the behavior between views.
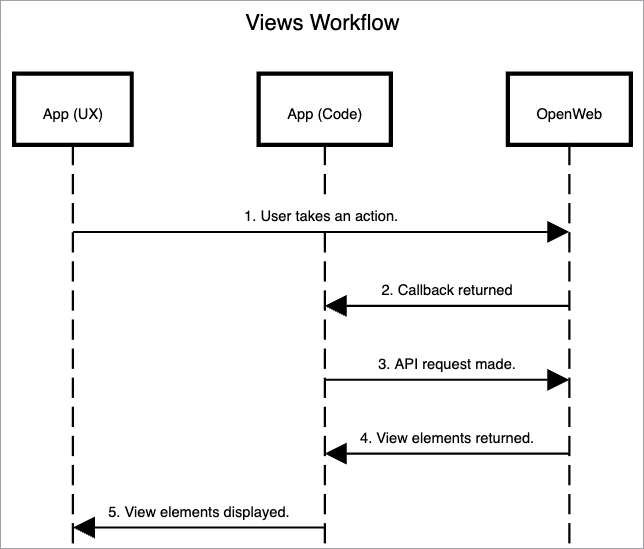
In this workflow, the following occurs:
- A user takes an action, such as tapping a button.
- The user's action triggers OpenWeb's callbacks API to return a callback, signifying an event occurred.
- As the developer, you use this event to make another API call to OpenWeb.
- OpenWeb's API responds with the specific view element related to the API call.
- As the developer, you manage the presentation of the views or elements returned by OpenWeb. You can decide whether these views or elements are displayed or hidden from the user.
The following table lists the configurable views that are returned.
View | Description |
---|---|
PreConversation | A preview of comments at the end of an article to encourage engagement with a Conversation |
Conversation | The main view for the Conversation |
Comment Creation | A view for entering a comment |
Comment Thread | Show a comment thread, starting from the root comment (commentId ) |
Report Reason | Screen listing possible report options
Use the OWViewActionsCallbacks to signal when to display these report options.
|
View Recipes
Use the following recipes to configure one of the views.
iOS SDK PreConversation View
Use async-await
async-await
Use an initializer or builder
iOS SDK Conversation View
Use async-await
async-await
Use an initializer or builder
iOS SDK Comment Creation View
Use async-await
async-await
Use an initializer or builder
iOS SDK Comment Thread View
Use async-await
async-await
Use a callback
iOS SDK Report Reason View
Use async-await
async-await
Use a callback
Methods
The following sections explain the methods available for flows and views.
commentCreation()
Starts the flow or retrieves the view for comment creation
var manager: OWManagerProtocol = OpenWeb.manager
// Retrieve flows layer
let flows: OWUIFlows = manager.ui.flows
flows.commentCreation(
postId: "somePostId",
article: article,
presentationalMode: OWModelPresentationStyle,
additionalSettings: commentCreationSettings,
completion: commentClosure
)
var manager: OWManagerProtocol = OpenWeb.manager
// Retrieve views layer
let views: OWUIViews = manager.ui.views
views.commentCreation(
postId: "somePostId",
additionalSettings: additionalSettings,
callbacks: OWViewActionsCallbacks,
completion: commentCreationViewClosure
)
commentThread()
Starts the flow or retrieves the view for comment thread
var manager: OWManagerProtocol = OpenWeb.manager
// Retrieve flows layer
let flows: OWUIFlows = manager.ui.flows
flows.commentThread(
postId: "somePostId",
article: article,
commentId: "someCommentId",
presentationalMode: .push(navigationController: navController),
additionalSettings: additionalSettings,
callbacks: nil,
completion: commentThreadClosure
)
var manager: OWManagerProtocol = OpenWeb.manager
// Retrieve views layer
let views: OWUIViews = manager.ui.views
views.commentCreation(
postId: "somePostId",
article: article
commentId: "someCommentId"
additionalSettings: additionalSettings,
callbacks: OWViewActionsCallbacks,
completion: commentCreationViewClosure
)
conversation()
Starts the flow or retrieves the view for the conversation
var manager: OWManagerProtocol = OpenWeb.manager
// Retrieve flows layer
let flows: OWUIFlows = manager.ui.flows
flows.conversation(
postId: "somePostId",
article: article,
presentationalMode: OWModelPresentationStyle,
additionalSettings: conversationSettings,
completion: conversationClosure
)
var manager: OWManagerProtocol = OpenWeb.manager
// Retrieve views layer
let views: OWUIViews = manager.ui.views
views.conversation(
postId: "somePostId",
article: article,
additionalSettings: additionalSettings,
callbacks: OWViewActionsCallbacks,
completion: conversationViewClosure
)
preConversation()
Starts the flow or retrieves the view for the pre-conversation
var manager: OWManagerProtocol = OpenWeb.manager
// Retrieve flows layer
let flows: OWUIFlows = manager.ui.flows
flows.preConversation(
postId: "somePostId",
article: article,
presentationalMode: OWModelPresentationStyle,
additionalSettings: preConversationSettings,
completion: preConversationClosure
)
var manager: OWManagerProtocol = OpenWeb.manager
// Retrieve views layer
let views: OWUIViews = manager.ui.views
views.preConversation(
postId: "somePostId",
article: article,
additionalSettings: additionalSettings,
callbacks: OWViewActionsCallbacks,
completion: preConversationViewClosure
)
reportReason()
Retrieves the view for the report reason
var manager: OWManagerProtocol = OpenWeb.manager
// Retrieve views layer
let views: OWUIViews = manager.ui.views
views.reportReason(
postId: "targetPostId",
commentId: "targetCommentId",
parentId: "parentCommentId",
additionalSettings: additionalSettings,
callbacks: reportActionsCallbacks,
completion: reportViewClosure
)
Property | Description |
---|---|
additionalSettings OWAdditionalSettingsProtocol | Defines the settings of each component in a flow/view
Possible Values:
|
article OWArticle | Information about the article associated with the flow
See: Article |
callbacks OWViewActionsCallbacks | Handles the response to a user action after the action has occurred
See OWViewActionsCallbacks |
commentId string | Unique identifier of a comment |
completion function | Closure that handles the completion of the flow, success scenarios, and failure scenarios
The closure logic is defined in the relevant recipe. Possible Values:
|
parentId string | Unique identifier of the parent comment |
postId string | Unique article identifier that is specific to the article page
The ideal postId has the following characteristics:
|
presentationalMode | Possible Values:
|
Enumerations and Structs
OWAdditionalSettings
public protocol OWAdditionalSettingsProtocol {
var preConversationSettings: OWPreConversationSettingsProtocol { get }
var fullConversationSettings: OWConversationSettingsProtocol { get }
var commentCreationSettings: OWCommentCreationSettingsProtocol { get }
var commentThreadSettings: OWCommentThreadSettingsProtocol { get }
}
Settings | Description |
---|---|
commentCreationSettings OWCommentCreationSettingsProtocol | Sets the initial comment creation flow settings
Possible Values:
|
fullconversationSettings OWConversationSettingsProtocol | Sets the initial Conversation flow settings
Possible Values:
|
preConversationSettings OWPreConversationSettingsProtocol | Sets the initial preConversation flow settings
Possible Values:
|
OWCommunityGuidelinesStyle
Setting | Description |
---|---|
OWCommunityGuidelinesStyle OWCommunityGuidelinesStyle | Manner in which the screen is displayed
Possible Values:
|
OWCommunityQuestionsStyle
Setting | Description |
---|---|
OWCommunityQuestionsStyle OWCommunityQuestionsStyle | Manner in which the screen is displayed
Possible Values:
|
OWConversationSpacing
Setting | Description |
---|---|
OWConversationSpacing OWConversationSpacing | Manner in which the screen is displayed
Possible Values:
|
OWError
Setting | Description |
---|---|
OWError string | Error returned |
OWFlowActionsCallbacks
typealias OWFlowActionsCallbacks = (
OWFlowActionCallbackType,
OWViewSourceType,
String)
-> Void
Settings | Description |
---|---|
OWFlowActionsCallbackType string | User action performed or error that occurred
See OWFlowActionCallbackType |
OWViewSourceType string | View from which information is received
See OWViewSourceType |
OWFlowActionCallbackType
Settings | Description |
---|---|
OWFlowActionCallbackType string | User action performed
Possible Values:
|
OWModalPresentationStyle
Setting | Description |
---|---|
OWModalPresentationStyle string | Manner in which the screen is displayed
Possible Values:
|
OWPresentationalMode
Setting | Description |
---|---|
OWPresentationalMode string | Manner in which the OpenWeb view is presented or pushed
Possible Values:
|
OWViewActionsCallbacks
typealias OWViewActionsCallbacks = (
OWViewActionCallbackType,
OWViewSourceType,
String
) -> Void
Settings | Description |
---|---|
OWViewActionCallbackType string | User action performed or error that occurred
See OWViewActionCallbackType |
OWViewSourceType string | View from which information is received
See OWViewSourceType |
OWViewActionCallbackType
Settings | Description |
---|---|
OWViewActionCallbackType string | User action performed or error that occurred
Possible Values:
|
OWViewCompletion
typealias OWViewCompletion = (
Result<UIView,
OWError>
) -> Void
Argument | Description |
---|---|
OWError string | Error returned
See: OWError
|
OWViewSourceType
Settings | Description |
---|---|
OWViewSourceType string | View from which information is received
Possible Values:
|
Updated 2 months ago