Customizations
With the OpenWeb SDK's OWCustomizations
protocol, you can craft a unique, unified appearance that aligns with your brand's identity.
Through OWCustomizations
, you can achieve the following:
- Modify the sort settings of comments through the
OWSortingCustomizations
protocol or aligning sort option names with specific branding terminology - Adapt your app's appearance through the theme settings of the
OWTheme
- Tailor UI elements, such as headers and navigation, through the
OWCustomizableElementCallback
callback
public protocol OWCustomizations {
var fontFamily: OWFontGroupFamily { get set }
var sorting: OWSortingCustomizations { get }
var themeEnforcement: OWThemeStyleEnforcement { get set }
var statusBarEnforcement: OWStatusBarEnforcement { get set }
var navigationBarEnforcement: OWNavigationBarEnforcement { get set }
var customizedTheme: OWTheme { get set }
func addElementCallback(_ callback: @escaping OWCustomizableElementCallback)
}
The following sections explain how to configure and define these settings.
Customization Settings
Customized Theme
var manager: OWManagerProtocol = OpenWeb.manager
var customizations: OWCustomizations = manager.ui.customizations
customizations.customizedTheme = OWTheme
Property | Description |
---|---|
OWTheme OWTheme | Enables granular theme customization
See: OWTheme
|
OWTheme
Defines the color to use for UI items in light (lightColor
) and dark (darkColor
) modes
When customizing an
OWTheme
property, bothlightColor
anddarkColor
must be defined.
OWTheme(
skeletonColor: OWColor(lightColor: UIColor, darkColor: UIColor),
skeletonShimmeringColor: OWColor(lightColor: UIColor, darkColor: UIColor),
primarySeparatorColor: OWColor(lightColor: UIColor, darkColor: UIColor),
secondarySeparatorColor: OWColor(lightColor: UIColor, darkColor: UIColor),
tertiarySeparatorColor: OWColor(lightColor: UIColor, darkColor: UIColor),
primaryTextColor: OWColor(lightColor: UIColor, darkColor: UIColor),
secondaryTextColor: OWColor(lightColor: UIColor, darkColor: UIColor),
tertiaryTextColor: OWColor(lightColor: UIColor, darkColor: UIColor),
primaryBackgroundColor: OWColor(lightColor: UIColor, darkColor: UIColor),
secondaryBackgroundColor: OWColor(lightColor: UIColor, darkColor: UIColor),
primaryBorderColor: OWColor(lightColor: UIColor, darkColor: UIColor),
loaderColor: OWColor(lightColor: UIColor, darkColor: UIColor),
brandColor: OWColor(lightColor: UIColor, darkColor: UIColor)
)
Background Color
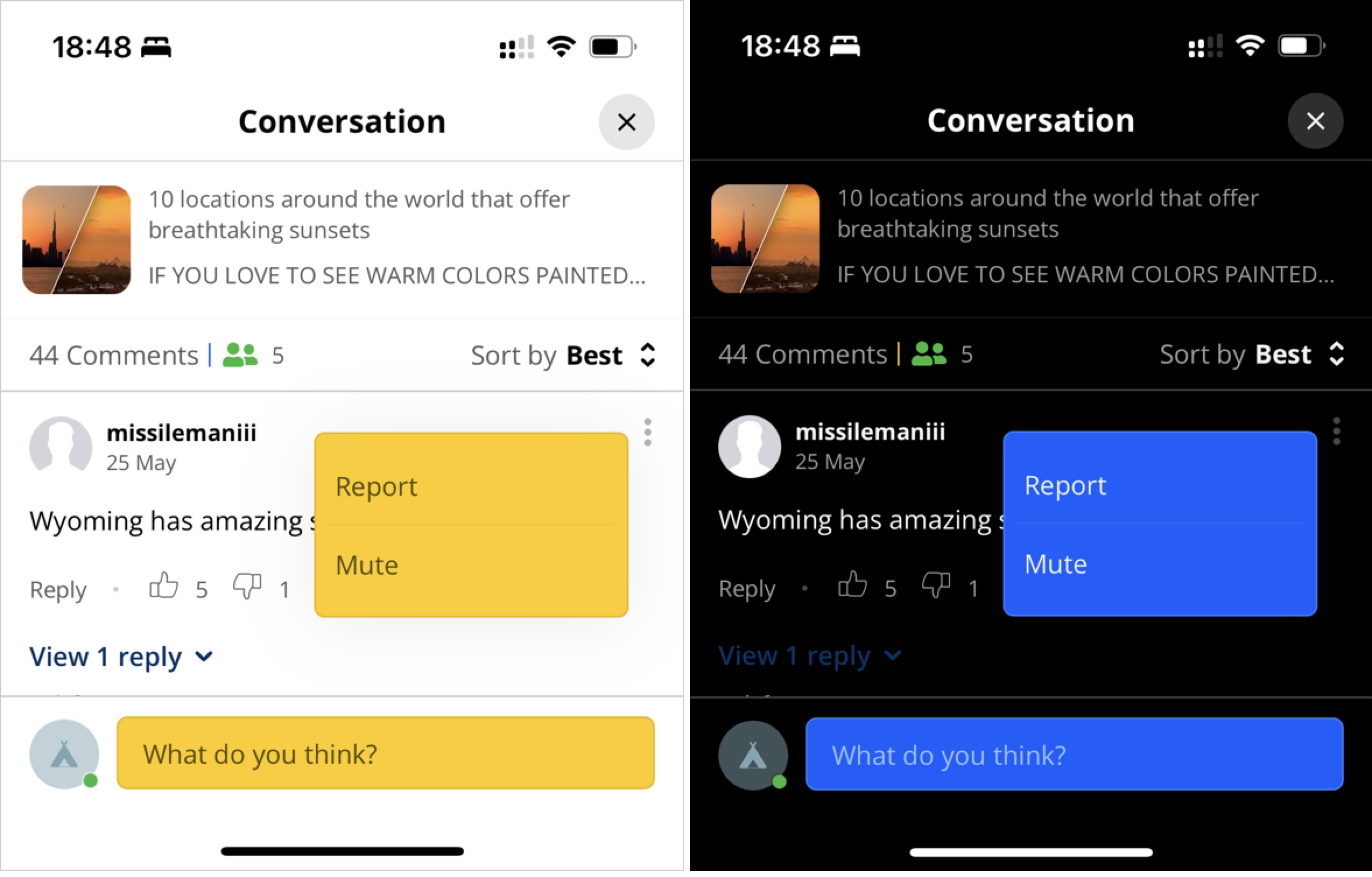
Light and dark theme background color customizations
OWTheme(
primaryBackgroundColor: OWColor(lightColor: .white, darkColor: .black),
secondaryBackgroundColor: OWColor(lightColor: .yellow, darkColor: .blue)
)
Property | Description |
---|---|
primaryBackgroundColor | Background color of the entire Conversation |
secondaryBackgroundColor | Background color of secondary items
|
Borders
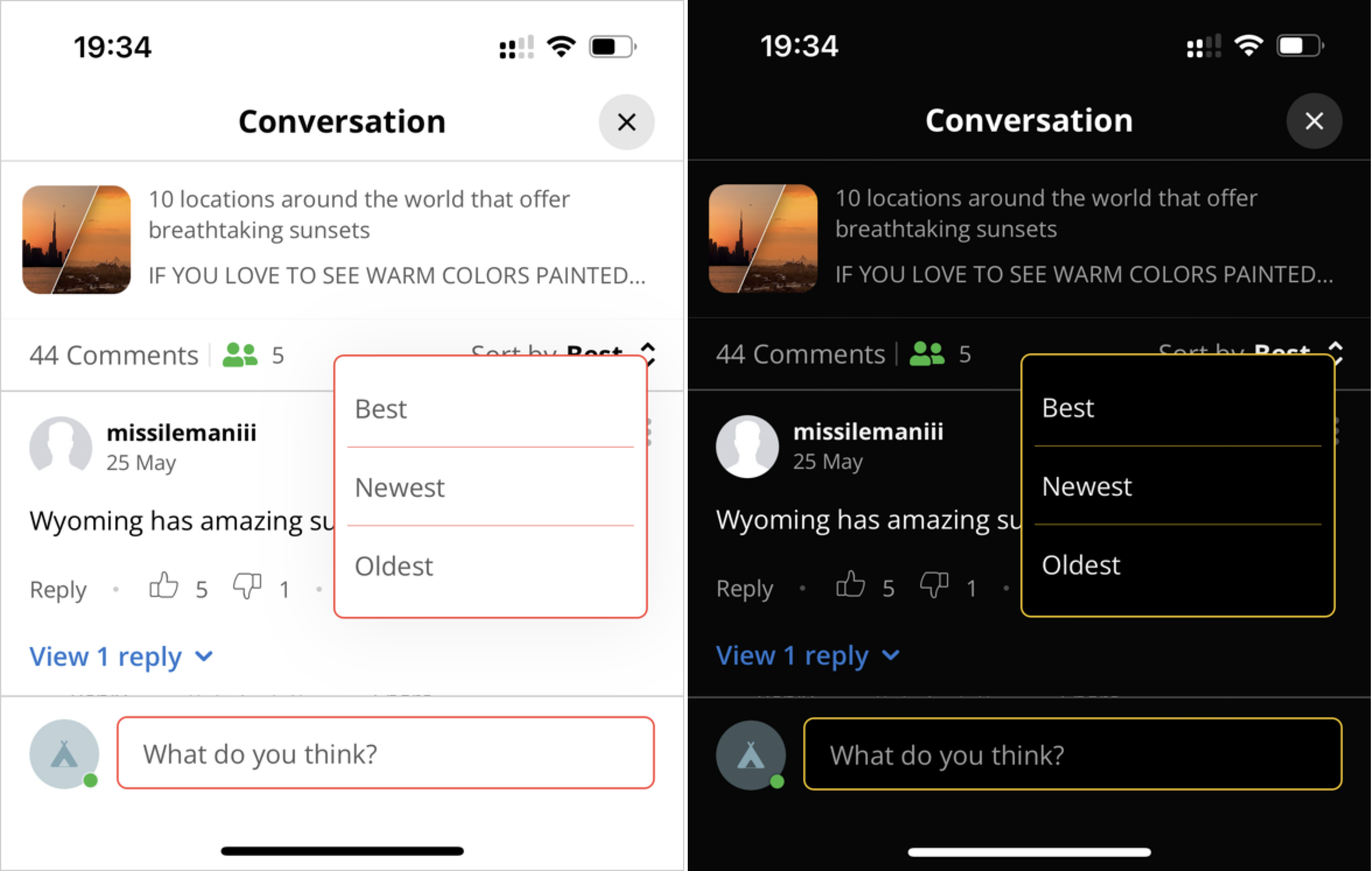
Light and dark theme border customizations
OWTheme(
primaryBorderColor: OWColor(lightColor: .red, darkColor: .yellow)
)
Property | Description |
---|---|
primaryBorderColor | Primary border color of the following UI elements:
|
Brand
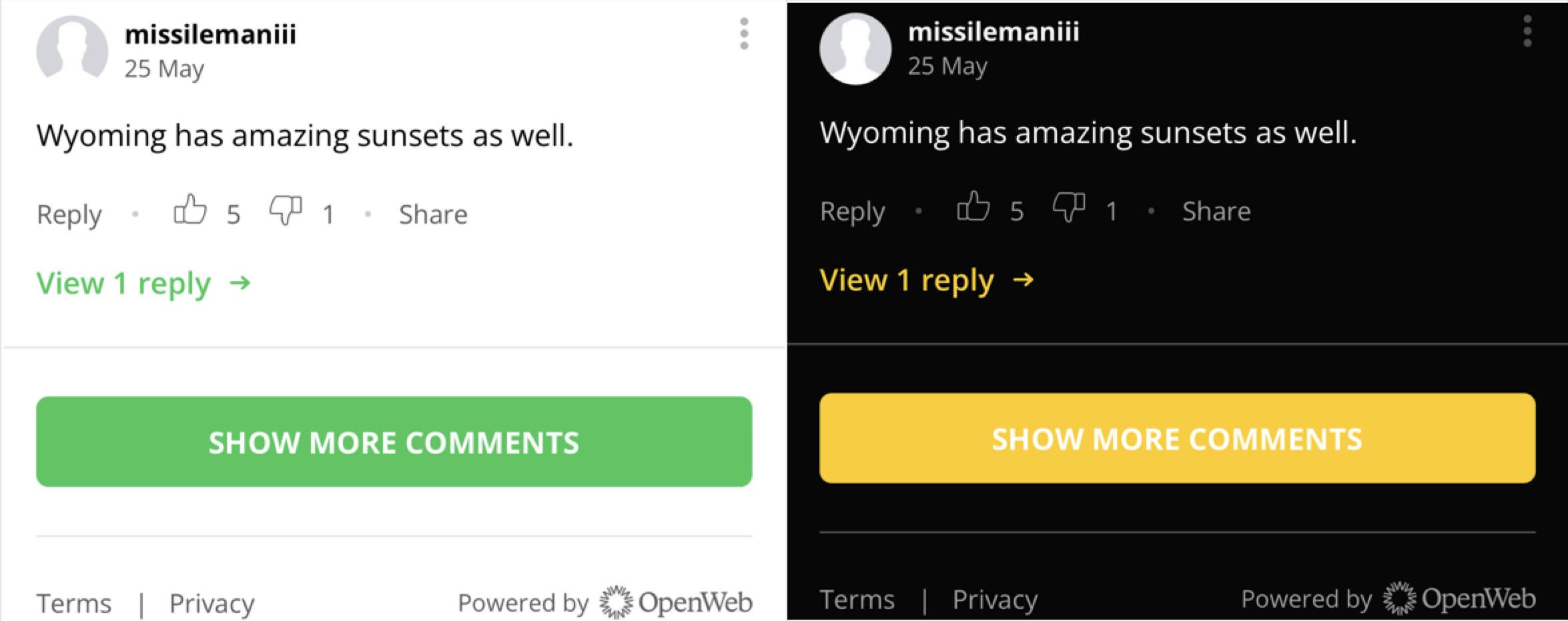
Light and dark theme brand color customization
OWTheme(
brandColor: OWColor(lightColor: .green, darkColor: .yellow)
)
Property | Description |
---|---|
brandColor | Primary color of the brand used in the following instances:
|
Loaders
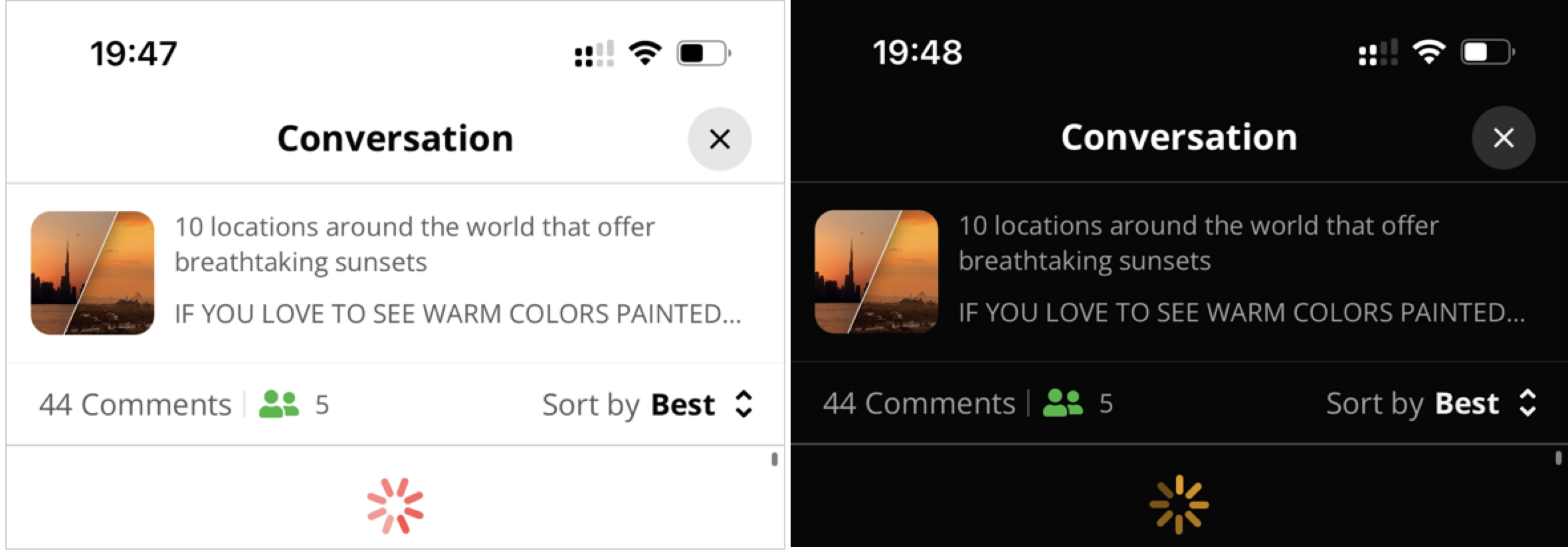
Light and dark theme loader color customization
OWTheme(
loaderColor: OWColor(lightColor: .red, darkColor: .yellow)
)
Property | Description |
---|---|
loaderColor | Color of the loading icon |
Separators
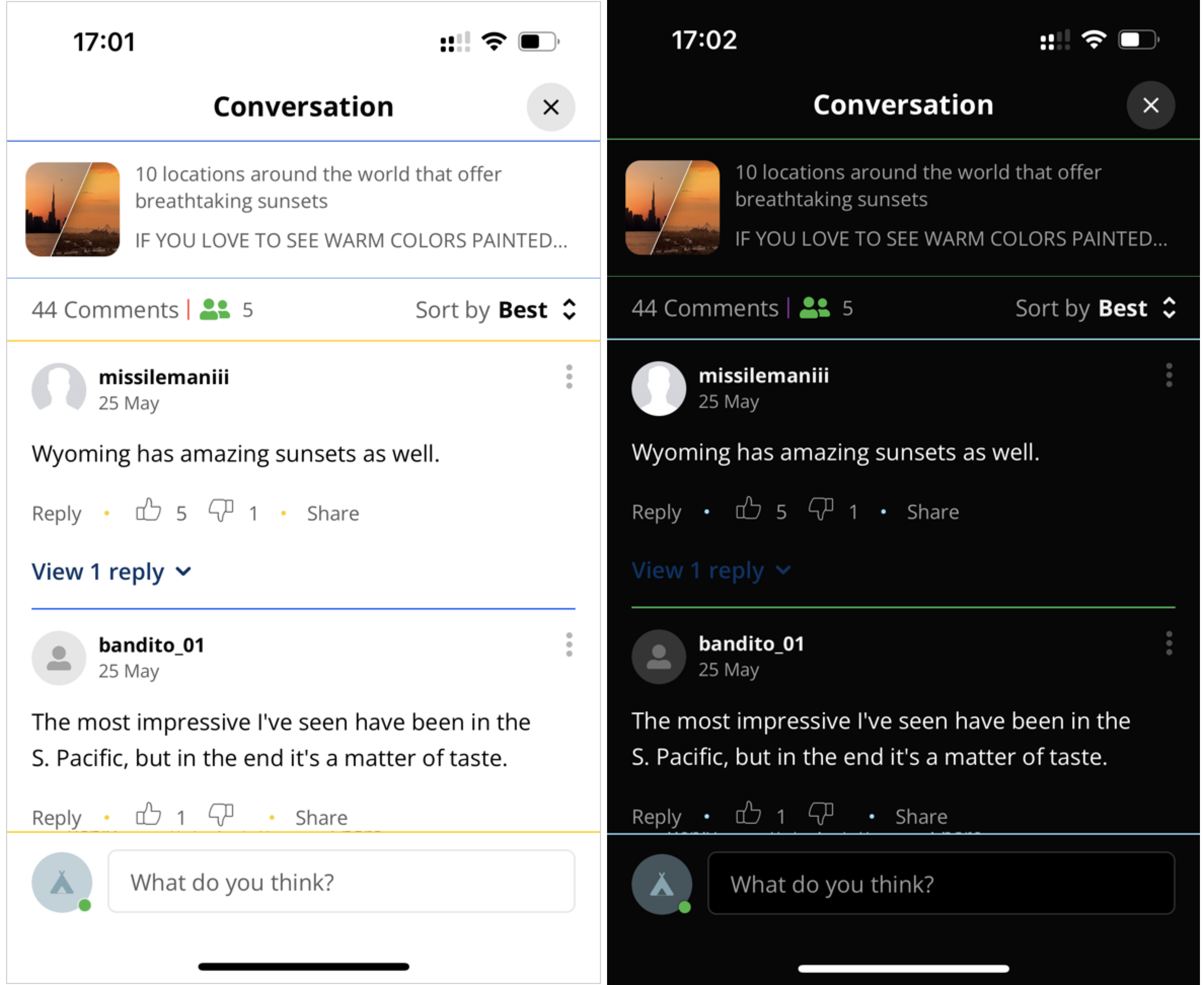
Light and dark theme separator customizations
OWTheme(
primarySeparatorColor: OWColor(lightColor: .blue, darkColor: .green),
secondarySeparatorColor: OWColor(lightColor: .red, darkColor: .purple),
tertiarySeparatorColor: OWColor(lightColor: .yellow, darkColor: .teal)
)
Property | Description |
---|---|
primarySeparatorColor | Color of the main separators:
|
secondarySeparatorColor | Color of pipe separator between the numbers of total comments and total commenters |
tertiarySeparatorColor | Color of the separators surrounding the comments pane:
|
Skeleton Color
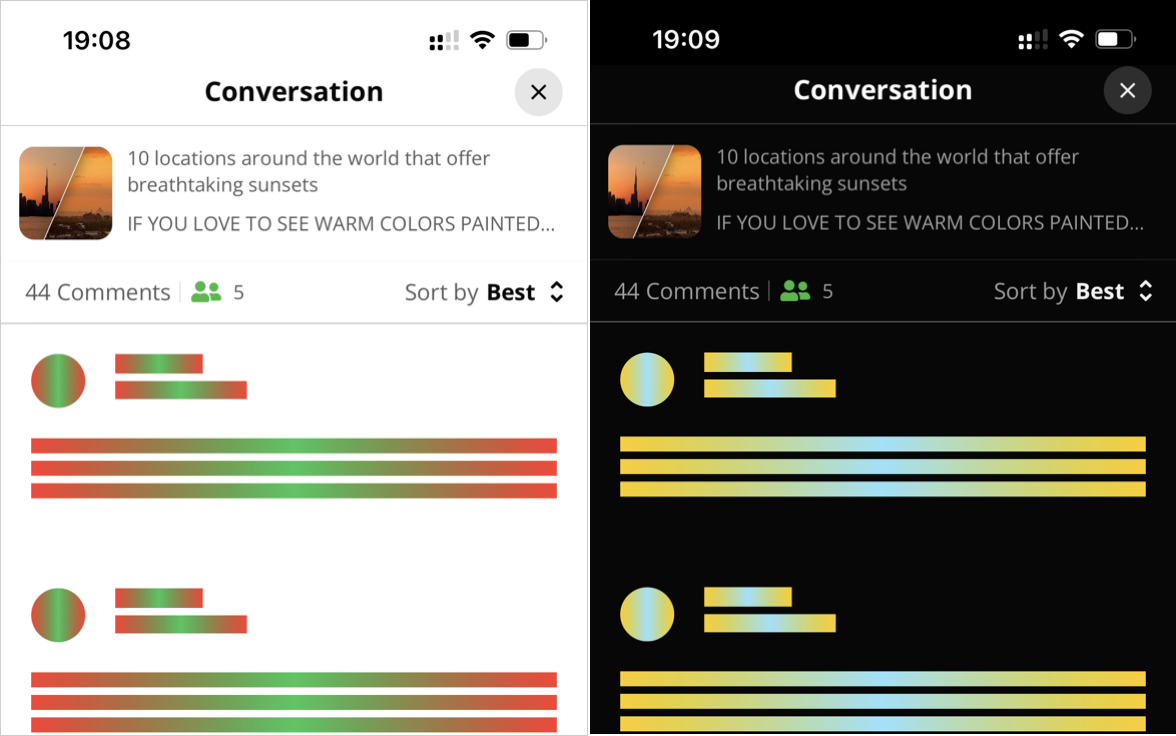
Light and dark theme skeleton customizations
OWTheme(
skeletonColor: OWColor(lightColor: .red, darkColor: .yellow),
skeletonShimmeringColor: OWColor(lightColor: .green, darkColor: .teal)
)
Property | Description |
---|---|
skeletonColor | Primary color of the UI element visual placeholder that appears while the UI element is loading |
skeletonShimmeringColor | Secondary color used to cause the illusion of movement and shimmering
Unlike the examples above, a gradient is applying to the color used for the skeletonColor with varying shades of a neutral color auch as gray. |
Text Color
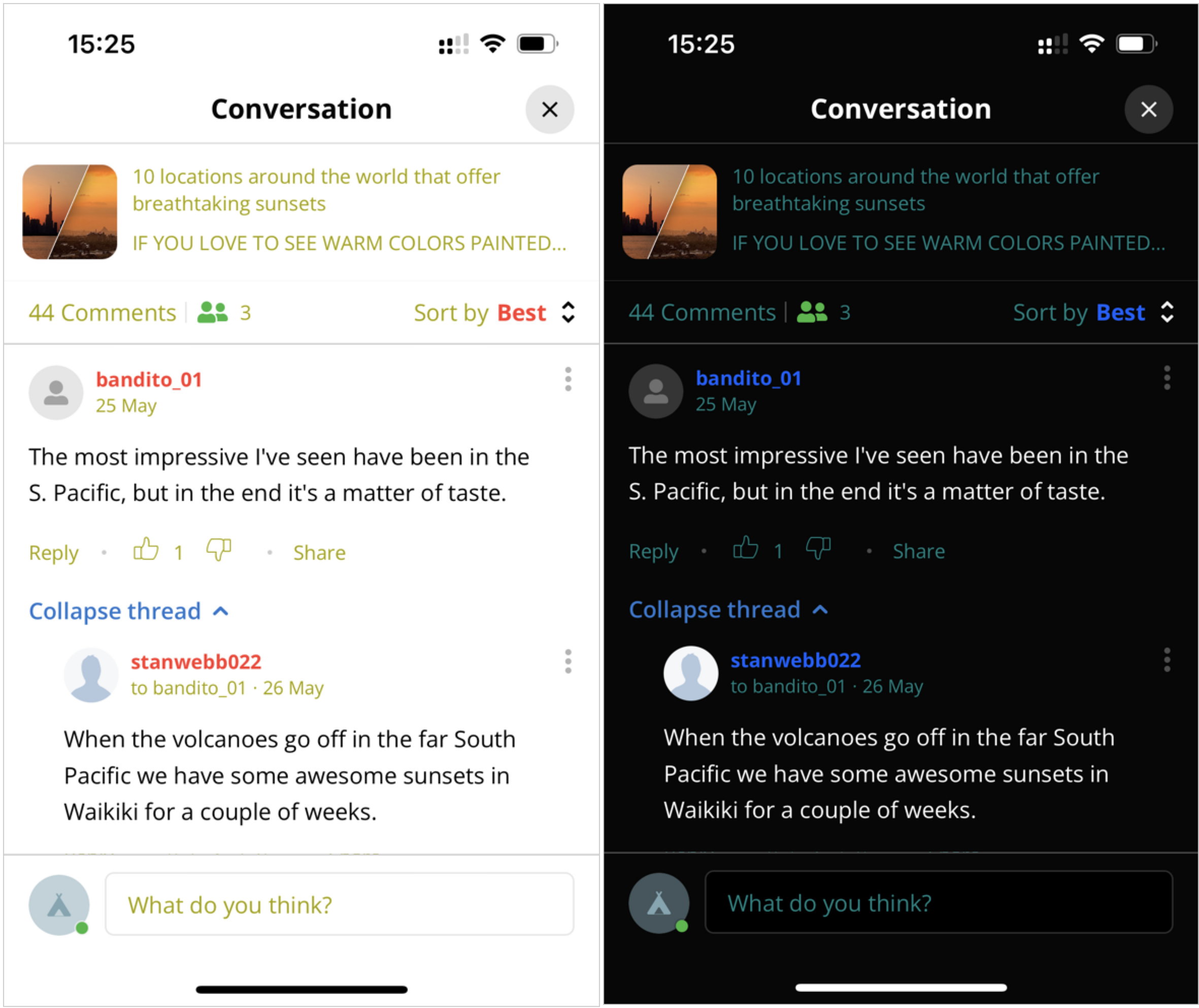
Light and dark theme text customizations
OWTheme(
primaryTextColor: OWColor(lightColor: .black, darkColor: .white),
secondaryTextColor: OWColor(lightColor: .red, darkColor: .blue),
tertiaryTextColor: OWColor(lightColor: .yellow, darkColor: .teal),
)
Property | Description |
---|---|
primaryTextColor | Color of message text |
secondaryTextColor | Color of username and selected Sort by option |
tertiaryTextColor | Color of other text items:
|
Fonts
var manager: OWManagerProtocol = OpenWeb.manager
var customizations: OWCustomizations = manager.ui.customizations
customizations.fontFamily = OWFontGroupFamily
Setting | Description |
---|---|
OWFontGroupFamily OWFontGroupFamily | Defines the UI font
See: OWFontGroupFamily
|
Navigation Bar Enforcement
var manager: OWManagerProtocol = OpenWeb.manager
var customizations: OWCustomizations = manager.ui.customizations
customizations.navigationBarEnforcement = OWNavigationBarEnforcement
Property | Description |
---|---|
OWNavigationBarEnforcement OWNavigationBarEnforcement | Defines the navigation bar appearance to enforce
See: OWNavigationBarEnforcement
|
Sorting
The OWSortingCustomizations
protocol defines the sort settings for the comments within the user interface.
var manager: OWManagerProtocol = OpenWeb.manager
var customizations: OWCustomizations = manager.ui.customizations
let sorting: OWSortingCustomizations = customizations.sorting
sorting.setTitle("BEST!!!" , forOption: .best)
sorting.initialOption = .use(sortOption:.newest)
initialOption
Defines the initial sorting of comments
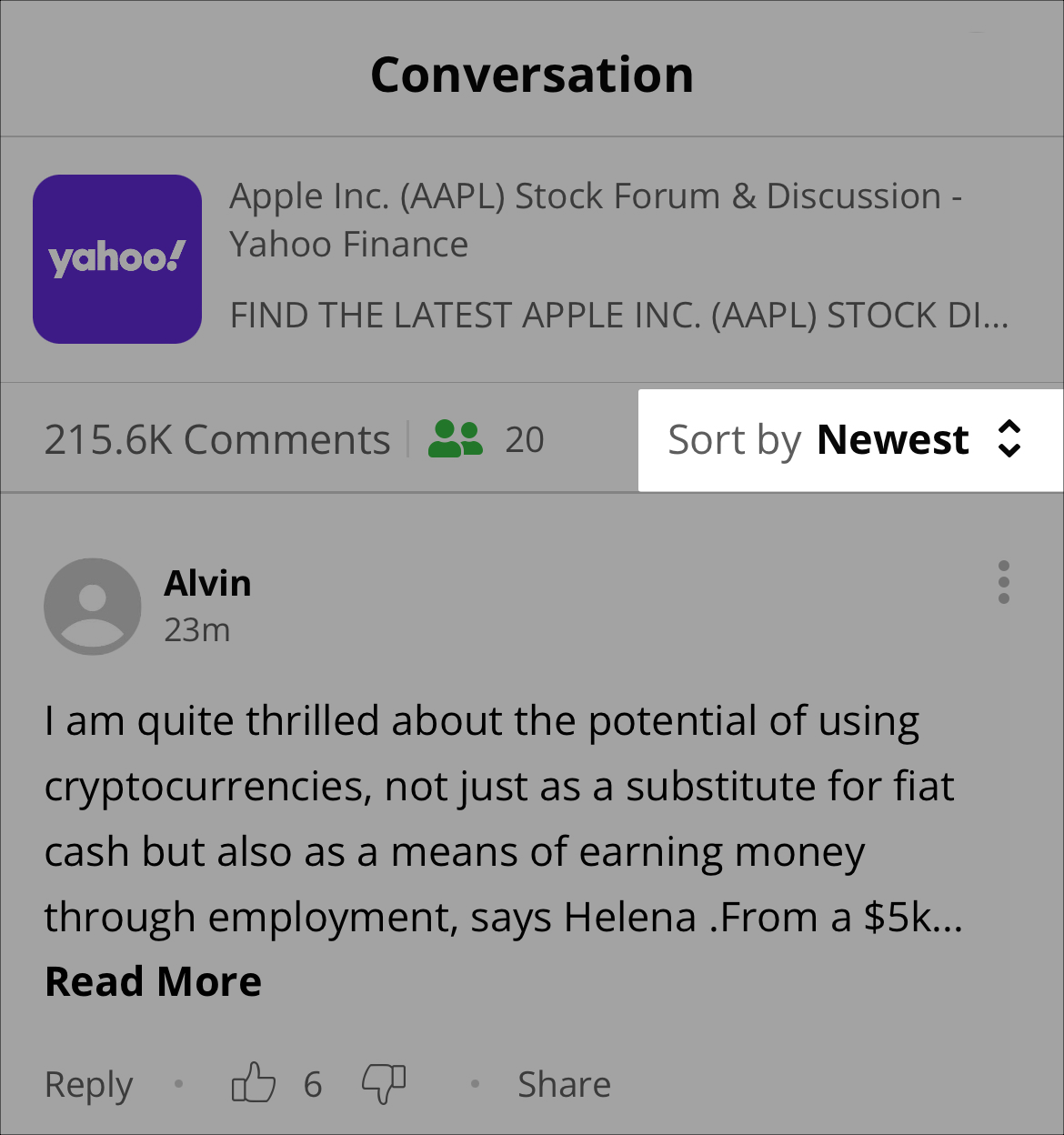
sorting.initialOption = .use(sortOption: OWSortOption)
Setting | Description |
---|---|
.use(sortOption: OWSortOption) | Permits defining the initial sort order of the comments shown in the user interface |
.useServerConfig | Uses the sort order defined in the Admin Panel |
setTitle()
Assigns a custom name to an available sorting option
This method enables you to align sort option names with your branding or specific regional wording.
setTitle(_ title: String , forOption option: OWSortOption)
Argument | Description |
---|---|
title | Custom name for the sort option
Example: "BEST!!!" |
option | Sort order option to which the custom name is applied |
Status Bar Enforcement
When customizing the status bar style, the following steps must be completed prior to theme enforcement:
- In the info.plist file, set View controller-based status bar appearance to YES.

info.plist settings
- To support status bar style customization when
presentionalMode: .push(navigationController: UINavigationController)
is set in a flows approach, subclassUINavigationController
and incorporate the following code.class HostApplicationNavigationController: UINavigationController { override var preferredStatusBarStyle: UIStatusBarStyle { return topViewController?.preferredStatusBarStyle ?? .default } }
var manager: OWManagerProtocol = OpenWeb.manager
var customizations: OWCustomizations = manager.ui.customizations
customizations.statusBarEnforcement = OWStatusBarEnforcement
Property | Description |
---|---|
OWStatusBarEnforcement OWStatusBarEnforcement | Defines the status bar appearance to enforce
See: OWStatusBarEnforcement
|
Theme Enforcement
var manager: OWManagerProtocol = OpenWeb.manager
var customizations: OWCustomizations = manager.ui.customizations
customizations.themeEnforcement = OWThemeStyleEnforcement
Property | Description |
---|---|
OWThemeStyleEnforcement OWThemeStyleEnforcement | Enforces either dark or light mode for the entire SDK, regardless of the device's active theme mode
See: OWThemeStyleEnforcement
|
UI Customization
The SDK allows you to customize UI elements.
var manager: OWManagerProtocol = OpenWeb.manager
var customizations: OWCustomizations = manager.ui.customizations
let customizableClosure: OWCustomizableElementCallback = { element, source, style, postId in
switch element {
case .communityQuestion(let textView):
// Do your customizations to the text view
case .default:
break
}
}
customizations.addElementCallback(customizableClosure) // Allow multiple callbacks
Setting | Description |
---|---|
OWCustomizableElementCallback OWCustomizableElementCallback | Callback for defining a user interface component to customize
See: OWCustomizableElementCallback |
Enumerations
OWCustomizableElement
Setting | Description |
---|---|
OWCustomizableElement OWCustomizableElement | UI component to customize
Possible Values:
|
OWArticleDescriptionCustomizableElement
Setting | Description |
---|---|
OWArticleDescriptionCustomizableElement OWHeaderCustomizableElement | Options for customization
Possible Values:
|
OWCommentCreationCTACustomizableElement
Setting | Description |
---|---|
OWCommentCreationCTACustomizableElement OWCommentCreationCTACustomizableElement | Options for customization
Possible Values:
|
OWCommentingEndedCustomizableElement
Setting | Description |
---|---|
OWCommentingEndedCustomizableElement OWCommentingEndedCustomizableElement | Options for customization
Possible Values:
|
OWCommunityGuidelinesCustomizableElement
Setting | Description |
---|---|
OWCommunityGuidelinesCustomizableElement OWCommunityGuidelinesCustomizableElement | Options for customization
Possible Values:
|
OWCommunityQuestionCustomizableElement
Setting | Description |
---|---|
OWCommunityQuestionCustomizableElement OWCommunityQuestionCustomizableElement | Options for customization
Possible Values:
|
OWEmptyStateCommentingEndedCustomizableElement
Setting | Description |
---|---|
OWEmptyStateCommentingEndedCustomizableElement OWEmptyStateCommentingEndedCustomizableElement | Options for customization
Possible Values:
|
OWEmptyStateCustomizableElement
Setting | Description |
---|---|
OWEmptyStateCustomizableElement OWEmptyStateCustomizableElement | Options for customization
Possible Values:
|
OWHeaderCustomizableElement
Setting | Description |
---|---|
OWHeaderCustomizableElement OWHeaderCustomizableElement | Options for customization
Possible Values:
|
OWLoginPromptCustomizableElement
Setting | Description |
---|---|
OWLoginPromptCustomizableElement OWNavigationCustomizableElement | Options for customizing the login prompt
![]() Possible Values:
|
OWNavigationCustomizableElement
Setting | Description |
---|---|
OWNavigationCustomizableElement OWNavigationCustomizableElement | Options for customization
Possible Values:
|
OWOnlineUsersCustomizableElement
Setting | Description |
---|---|
OWOnlineUsersCustomizableElement OWSummaryHeaderCustomizableElement | Options for customization
Possible Values:
|
OWSummaryCustomizableElement
Setting | Description |
---|---|
OWSummaryCustomizableElement OWSummaryCustomizableElement | Options for customization
Possible Values:
|
OWSummaryHeaderCustomizableElement
Setting | Description |
---|---|
OWSummaryHeaderCustomizableElement OWSummaryHeaderCustomizableElement | Options for customization
Possible Values:
|
OWCustomizableElementCallback
typealias OWCustomizableElementCallback = (
element: OWCustomizableElement,
sourceType: OWViewSourceType,
style: OWThemeStyle,
postId: String?
) -> Void
customizations.addElementCallback(customizableClosure)
Argument | Description |
---|---|
element OWCustomizableElement | User interface component to customize
See: OWCustomizableElement |
postId string | Unique article identifier that is specific to the article page
The ideal postId has the following characteristics:
|
sourceType OWViewSourceType | Location of the user interface element
See: OWViewSourceType |
style OWThemeStyle | Theme to apply to the element
See: OWThemeStyle |
OWFontGroupFamily
Setting | Description |
---|---|
OWFontGroupFamily OWFontGroupFamily | Options to set the UI font
Possible Values:
|
OWNavigationBarEnforcement
Setting | Description |
---|---|
OWNavigationBarEnforcement OWNavigationBarEnforcement | Style of navigation bar to enforce
Possible Values:
|
OWNavigationBarStyle
Setting | Description |
---|---|
OWNavigationBarStyle OWNavigationBarStyle | Navigation bar style options
Possible Values:
|
OWSortOption
Setting | Description |
---|---|
OWSortOption string | Options for sorting comments
Possible Values:
|
OWStatusBarEnforcement
Setting | Description |
---|---|
OWStatusBarEnforcement OWStatusBarEnforcement | Style of status bar to enforce
Possible Values:
|
OWThemeStyle
Setting | Description |
---|---|
OWThemeStyle string | Options for theme styles
Possible Values:
|
OWThemeStyleEnforcement
customizations.themeEnforcement = .theme(_ theme: OWThemeStyle)
Property | Description |
---|---|
.none | No theme style is enforced |
.theme(_ theme: OWThemeStyle) | Sets the theme style that is enforced
See: OWThemeStyle
|
OWViewSourceType
Setting | Description |
---|---|
OWViewSourceType string | Options for a user interface location when customizing UI elements
Possible Values:
|
Updated 3 days ago