Customization
The Customizations section of the OpenWeb Android SDK empowers developers to tailor the user interface and interaction elements to align with their brand identity and user experience goals.
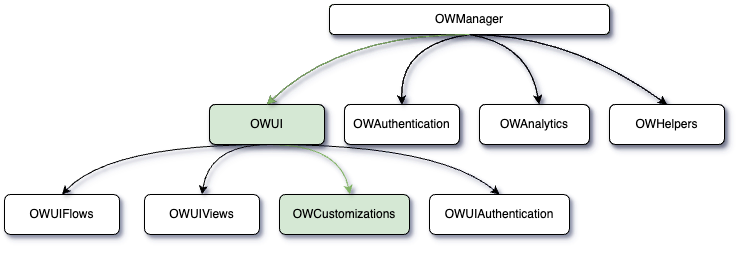
By leveraging the customization APIs, publishers can:
- Define sorting behaviors and labels for comment threads.
- Control the theme (light, dark, or custom) to match the application's aesthetic.
- Customize the appearance of comment actions, such as button colors and fonts.
- Add accessory views to extend or enhance predefined UI components.
- Integrate Giphy for engaging gif selections within comments.
Customizations are handled through a set of structured APIs under the OWCustomizations interface, offering flexibility and ease of integration without compromising the core functionality of the SDK.
This section guides you through key customization capabilities, with examples and references to ensure seamless implementation.
Sorting
Sorting options let you customize the initial sorting order and labels for comment threads.
-
initialSortOption: Specifies the default sorting order. Possible values include:
BEST
: (Default).NEWEST
OLDEST
-
setTitle(title: String, sortOption: OWSortOption): Assigns a custom title for a sort option.
Example:
val sortingCustomizations: OWSortingCustomizations = OpenWeb.manager.ui.customizations.sorting
// Set the default sorting option to NEWEST
sortingCustomizations.initialSortOption = OWSortOption.NEWEST
// Customize the title for "BEST"
sortingCustomizations.setTitle("Top Comments", OWSortOption.BEST)
Theme
With the OpenWeb SDK, you can define light, dark, or fully customized themes to match your application's design.
Theme Enforcement
Theme Enforcement allows developers to set the theme mode and customize the color scheme for dark mode.
-
themeMode: Defines the theme mode. Options include:
LIGHT
(Default)DARK
-
darkColor: Allows setting a custom dark color scheme.
Example:
val customizations = OpenWeb.manager.ui.customizations
// Enable dark mode
customizations.themeEnforcement.themeMode = OWThemeMode.DARK
Customized Theme
Customized Theme provides complete control over colors for both light and dark modes. Developers can specify colors for various UI components using the OWTheme class.
Warning
When customizing an OWTheme property, both
lightColor
anddarkColor
must be defined.
Example:
customizations.customizedTheme = OWTheme(
primaryBackgroundColor = UIColor(0xFF000000),
secondaryTextColor = UIColor(0xFF888888),
brandColor = UIColor(0xFFFF5722)
)
Property | Description |
---|---|
skeletonColor | Color for loading skeletons. |
skeletonShimmeringColor | Shimmer effect color for loading skeletons. |
primarySeparatorColor | Primary separator line color. |
secondarySeparatorColor | Secondary separator line color. |
tertiarySeparatorColor | Tertiary separator line color. |
primaryTextColor | Primary text color for main content. |
secondaryTextColor | Secondary text color for supporting content. |
tertiaryTextColor | Tertiary text color for less prominent content. |
primaryBackgroundColor | Background color for primary views. |
secondaryBackgroundColor | Background color for secondary views. |
tertiaryBackgroundColor | Background color for tertiary views. |
primaryBorderColor | Border color for primary elements. |
secondaryBorderColor | Border color for secondary elements. |
loaderColor | Color of loading indicators. |
brandColor | Brand's primary color. |
voteUpSelectedColor | Color for the "upvote" button when selected. |
voteUpUnselectedColor | Color for the "upvote" button when unselected. |
voteDownSelectedColor | Color for the "downvote" button when selected. |
voteDownUnselectedColor | Color for the "downvote" button when unselected. |
statusBarColor (deprecated) | Status bar color. Deprecated in API level 35; use primaryBackgroundColor . |
navigationBarColor (deprecated) | Navigation bar color. Deprecated in API level 35; use primaryBackgroundColor . |
Comment Action
Control the appearance of comment action buttons, such as their colors and fonts.
-
commentActionsButtonsColor: Options include:
DEFAULT
BRAND_COLOR
-
commentActionsButtonsFont: Options include:
DEFAULT
SEMI_BOLD
Example:
val customizations = OpenWeb.manager.ui.customizations
// Customize comment action buttons
customizations.commentActions = OWCommentActionsCustomizations(
commentActionsButtonsColor = CommentActionsButtonsColor.BRAND_COLOR,
commentActionsButtonsFont = CommentActionsButtonsFont.SEMI_BOLD
)
Font Family
Customize the font style used throughout the SDK's UI components.
- fontFamily: Represents a font style resource ID that can be applied globally to text elements in the SDK.
Example:
val customizations = OpenWeb.manager.ui.customizations
// Set a custom font family
customizations.fontFamily.styleResId = R.font.my_custom_font
Accessory Views
Extend or replace predefined UI elements using accessory views.
- setAccessoryViewProvider(viewProvider: OWAccessoryViewProvider): Registers a custom view provider for the SDK.
Example:
customizations.setAccessoryViewProvider(object : OWAccessoryViewProvider {
override fun provideView(
viewType: OWAccessoryViewType,
inflater: LayoutInflater,
parent: ViewGroup?
): View? {
if (viewType == OWAccessoryViewType.BottomToolbar) {
return inflater.inflate(R.layout.custom_toolbar, parent, false)
}
return null
}
})
Giphy Integration
Enable users to select and share GIFs using the Giphy SDK.
- setGiphyProvider(giphyProvider: SpotGiphyProvider): Connects the OpenWeb SDK with the Giphy SDK.
Example:
customizations.setGiphyProvider(object : SpotGiphyProvider {
override fun configure(activityContext: Context, sdkKey: String) {
// Giphy configuration logic
}
override fun showGiphyDialogFragment(
giphySetting: GiphySetting,
fragmentManager: FragmentManager,
fragmentTag: String,
selectionListener: GifSelectionListener
) {
// Show the Giphy dialog fragment
}
})
Custom UI Delegate
You can customize individual UI components by implementing a custom delegate.
- setCustomUIDelegate(customUIDelegate: CustomUIDelegate): Allows customization of specific views within the SDK.
Example:
customizations.setCustomUIDelegate(object : CustomUIDelegate {
override fun customizeView(
viewType: CustomizableViewType,
view: View,
isDarkModeEnabled: Boolean,
postId: OWPostId
) {
if (viewType == CustomizableViewType.NAVIGATION_TITLE_TEXT_VIEW) {
(view as? TextView)?.apply {
text = "Custom Navigation Title"
setTextColor(Color.RED)
}
}
}
})
View Type | Description |
---|---|
LOGIN_PROMPT_TEXT_VIEW | Customizes the login prompt text displayed for unauthenticated users. |
SAY_CONTROL_IN_PRE_CONVERSATION_TEXT_VIEW | Customizes the "Say something" control in the pre-conversation view. |
SAY_CONTROL_IN_CONVERSATION_TEXT_VIEW | Customizes the "Say something" control in the conversation view. |
CONVERSATION_FOOTER_VIEW | Customizes the footer section of the conversation. |
COMMUNITY_QUESTION_TEXT_VIEW | Customizes the community question text view. |
NAVIGATION_TITLE_TEXT_VIEW | Customizes the navigation title text. |
NAVIGATION_BACK_IMAGE_VIEW | Customizes the back button image in the navigation bar. |
NAVIGATION_TOOLBAR_VIEW | Customizes the entire navigation toolbar. |
COMMUNITY_GUIDELINES_TEXT_VIEW | Customizes the community guidelines text. |
SHOW_COMMENTS_BUTTON | Customizes the "Show Comments" button. |
PRE_CONVERSATION_HEADER_TEXT_VIEW | Customizes the header text in the pre-conversation view. |
PRE_CONVERSATION_HEADER_COUNTER_TEXT_VIEW | Customizes the counter text in the pre-conversation header. |
COMMENT_CREATION_ACTION_BUTTON | Customizes the action button for creating comments. |
COMMENT_CREATION_ACTION_IMAGE_BUTTON | Customizes the image button for creating comments. |
READ_ONLY_TEXT_VIEW | Customizes the text view displayed in read-only mode. |
EMPTY_STATE_READ_ONLY_TEXT_VIEW | Customizes the text view displayed when there are no comments in read-only mode. |
Navigation Bar Color
- setUseWhiteNavigationColor(useWhiteNavigationColor: Boolean): Configures the navigation bar color to white.
Example:
customizations.setUseWhiteNavigationColor(true)
For additional examples or advanced use cases, refer to the specific customization APIs in the reference section.
Updated about 1 month ago