GIPHY Integration for Android
Enable users to share animated GIFs from the GIPHY library within the Android SDK
The GIPHY library allows users to search a library of animated GIFs and share them in the conversation, adding a dynamic and visual element to the user experience.
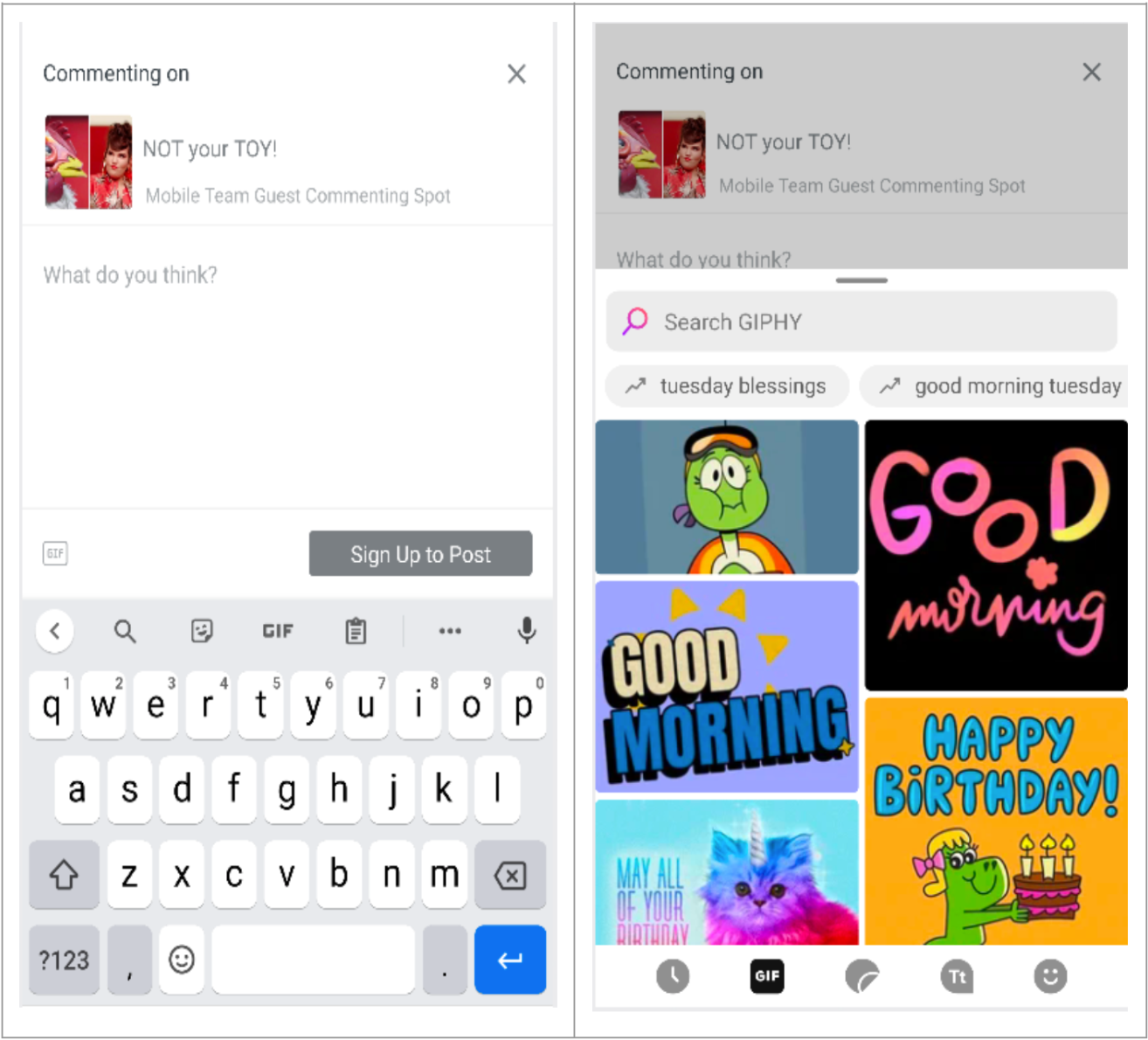
Leaving a Gif on a comment
Enable Giphy for your comments
To enable this feature, use the following steps to add the Giphy SDK dependency and implement the SpotGiphyProvider
:
- In the app module build.gradle flie, add the Giphy dependency.
implementation 'com.giphy.sdk:ui:2.1.12'
- Create a
GiphyHandler
class in the source code that implements theSpotGiphyProvider
.
import android.content.Context
import androidx.fragment.app.FragmentManager
import com.giphy.sdk.core.models.Image
import com.giphy.sdk.core.models.Media
import com.giphy.sdk.core.models.enums.RatingType
import com.giphy.sdk.ui.GPHContentType
import com.giphy.sdk.ui.GPHSettings
import com.giphy.sdk.ui.Giphy
import com.giphy.sdk.ui.themes.GPHTheme
import com.giphy.sdk.ui.views.GiphyDialogFragment
import spotIm.common.gif.*
class GiphyHandler: SpotGiphyProvider {
override fun configure(activityContext: Context, sdkKey: String) {
Giphy.configure(activityContext, sdkKey)
}
override fun showGiphyDialogFragment(
giphySetting: GiphySetting,
fragmentManager: FragmentManager,
fragmentTag: String,
selectionListener: GifSelectionListener
) {
val theme = when (giphySetting.theme) {
GiphyTheme.DARK -> GPHTheme.Dark
GiphyTheme.LIGHT -> GPHTheme.Light
}
val rating = when (giphySetting.rating) {
GiphyRating.Y -> RatingType.y
GiphyRating.G -> RatingType.g
GiphyRating.R -> RatingType.r
GiphyRating.PG -> RatingType.pg
GiphyRating.PG13 -> RatingType.pg13
}
val settings = GPHSettings(rating = rating, theme = theme)
val gifsDialog = GiphyDialogFragment.newInstance(settings)
gifsDialog.gifSelectionListener =
object : GiphyDialogFragment.GifSelectionListener {
private fun toGiphyImage(image: Image?): GiphyImage? {
return image?.let {
GiphyImage(it.gifUrl, it.webPUrl, it.height, it.width)
}
}
private fun toGiphyMedia(media: Media): GiphyMedia {
return GiphyMedia(
toGiphyImage(media.images.original),
toGiphyImage(media.images.preview)
)
}
override fun didSearchTerm(term: String) {
//Callback for search terms
}
override fun onDismissed(selectedContentType: GPHContentType) {
//Your user dismissed the dialog without selecting a GIF
}
override fun onGifSelected(
media: Media,
searchTerm: String?,
selectedContentType: GPHContentType
) {
selectionListener.onGifSelected(toGiphyMedia(media), searchTerm)
}
}
gifsDialog.show(fragmentManager, fragmentTag)
}
}
import android.content.Context;
import androidx.fragment.app.FragmentManager;
import com.giphy.sdk.core.models.Image;
import com.giphy.sdk.core.models.Media;
import com.giphy.sdk.core.models.enums.RatingType;
import com.giphy.sdk.ui.GPHContentType;
import com.giphy.sdk.ui.GPHSettings;
import com.giphy.sdk.ui.Giphy;
import com.giphy.sdk.ui.themes.GPHTheme;
import com.giphy.sdk.ui.views.GiphyDialogFragment;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import spotIm.common.gif.GifSelectionListener;
import spotIm.common.gif.GiphyImage;
import spotIm.common.gif.GiphyMedia;
import spotIm.common.gif.GiphySetting;
import spotIm.common.gif.SpotGiphyProvider;
public class GiphyHandler implements SpotGiphyProvider {
@Override
public void configure(@NotNull Context activityContext, @NotNull String sdkKey) {
Giphy.INSTANCE.configure(activityContext, sdkKey);
}
@Override
public void showGiphyDialogFragment(
@NotNull GiphySetting giphySetting,
@NotNull FragmentManager fragmentManager,
@NotNull String fragmentTag,
@NotNull GifSelectionListener selectionListener
) {
GPHTheme theme = GPHTheme.Light;
switch (giphySetting.getTheme()) {
case LIGHT:
theme = GPHTheme.Light;
break;
case DARK:
theme = GPHTheme.Dark;
break;
}
RatingType rating = RatingType.y;
switch (giphySetting.getRating()) {
case Y:
rating = RatingType.y;
break;
case G:
rating = RatingType.g;
break;
case R:
rating = RatingType.r;
break;
case PG:
rating = RatingType.pg;
break;
case PG13:
rating = RatingType.pg13;
break;
}
GPHSettings settings = new GPHSettings();
settings.setRating(rating);
settings.setTheme(theme);
GiphyDialogFragment gifsDialog = GiphyDialogFragment.Companion.newInstance(settings);
gifsDialog.setGifSelectionListener(new GiphyDialogFragment.GifSelectionListener() {
private GiphyImage toGiphyImage(Image image) {
return new GiphyImage(image.getGifUrl(), image.getWebPUrl(), image.getHeight(), image.getWidth());
}
private GiphyMedia toGiphyMedia(Media media) {
return new GiphyMedia(
toGiphyImage(media.getImages().getOriginal()),
toGiphyImage(media.getImages().getPreview())
);
}
@Override
public void onGifSelected(@NotNull Media media, @Nullable String searchTerm, @NotNull GPHContentType gphContentType) {
selectionListener.onGifSelected(toGiphyMedia(media), searchTerm);
}
@Override
public void onDismissed(@NotNull GPHContentType gphContentType) {
//Your user dismissed the dialog without selecting a GIF
}
@Override
public void didSearchTerm(@NotNull String s) {
//Callback for search terms
}
});
gifsDialog.show(fragmentManager, fragmentTag)
}
}
- Set the
GiphyHandler
toSpotIm
.
SpotIm.setGiphyProvider(giphyHandler)
SpotIm.setGiphyProvider(giphyHandler);
Updated over 1 year ago