AD UI
The OWIAUUI
protocol allows you to integrate and display ads in your application. This documentation provides an overview of the protocol, its associated types, and a guide for implementation.
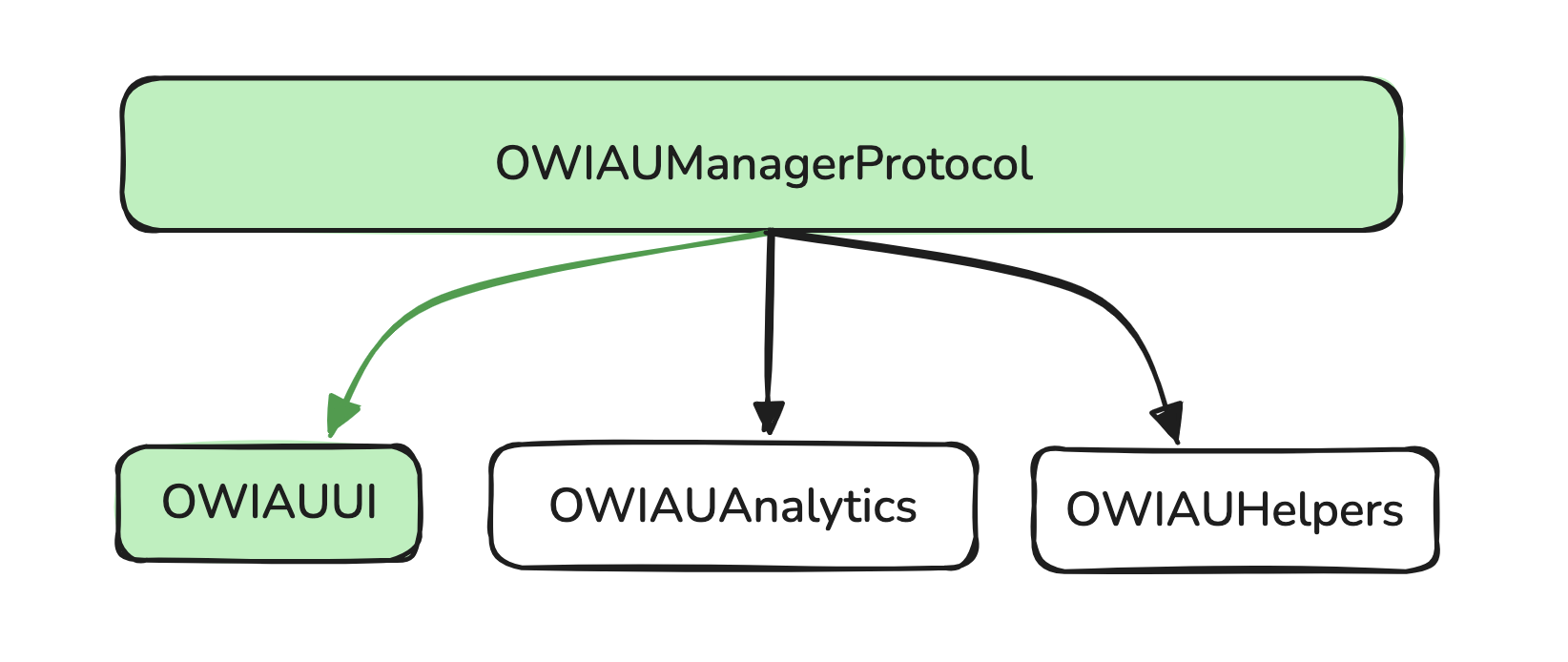
Display Ads Using the OWIAUUI Protocol
After obtaining tracking authorization, use the OWIAUUI
protocol to display the ad:
public protocol OWIAUUI {
func ad(postId: OWIAUPostId,
settings: OWIAUAdSettingsProtocol,
viewEventCallbacks: OWIAUAdViewEventsCallbacks?,
actionsCallbacks: OWIAUAdViewActionsCallbacks?,
completion: @escaping OWIAUViewCompletion)
}
To render the ad in the view:
import OpenWebIAUSDK
let adConfiguration = OWIAUAdConfiguration.server(remote: .tmsServer(index: 0))
let adSettings: OWIAUAdSettingsProtocol = OWIAUAdSettings(configuration: adConfiguration)
let viewEventCallbacks: OWIAUAdViewEventsCallbacks = { [weak self] eventType in
switch eventType {
case .video(let event):
// Handle the video view event
case .display(let event):
// Handle the display view event
}
}
let actionsCallbacks: OWIAUAdViewActionsCallbacks = { [weak self] event in
switch event {
case let .adSizeChanged(uiView, size):
// Note: Can manage height constrain from this event
default: break
}
}
manager.ui.ad(postId: "somePostId",
settings: adSettings,
viewEventCallbacks: viewEventCallbacks,
actionsCallbacks: actionsCallbacks,
completion: { [weak self] result in
guard let self else { return }
switch result {
case .success(let adView):
view.addSubview(adView)
/// <---- Add constraints
case .failure(let error):
print(error.localizedDescription)
}
})
Property Explanations:
PostID
: A unique identifier for the article page where the ad will be displayed.OWIAUAdSettings
: Provides the configuration for the ad.
public protocol OWIAUAdSettingsProtocol {
var configuration: OWIAUAdConfiguration { get }
}
public enum OWIAUAdConfiguration {
case server(remote: OWIAURemoteAdsStrategy)
}
public enum OWIAURemoteAdsStrategy {
case tmsServer(index: Int)
}
The index
will be used to get different configurations provided by PS.
OWIAUAdViewEventsCallbacks
public typealias OWIAUAdViewEventsCallbacks = (
OWIAUViewEventCallbackType,
OWIAUViewSourceType,
OWIAUPostId
) -> Void
public enum OWIAUViewEventCallbackVideoType {
case adServerCalled
case inventory
case viewableImpression
case sourceLoaded
case impression
case progress(_ progress: OWIAUVideoProgress)
case fullScreenToggleRequested
case movedToFullscreen
case movedFromFullscreen
case clickThrough
case skippableStateChange
case adPaused
case closed
case error(_ error: String)
case generic(_ name: String)
}
public enum OWIAUVideoProgress {
case started
case firstQuartile
case midpoint
case thirdQuartile
case complete
}
public enum OWIAUViewEventCallbackDisplayType {
case inventory
case sourceLoaded
case error(_ error: String)
case impression
case viewableImpression
case clickThrough
case closed
}
public enum OWIAUViewSourceType {
case ad
}
OWIAUAdViewActionsCallbacks
public typealias OWIAUAdViewActionsCallbacks = (OWIAUViewActionCallbackType, OWIAUViewSourceType, OWIAUPostId) -> Void
public enum OWIAUViewActionCallbackType {
case adSizeChanged(UIView, CGSize)
}
Completion Function
The completion function returns either a success scenario with the ad view or a failure scenario with an error.
By following these steps, you can easily integrate ads into your iOS app using the iOS IAU SDK, managing the ad configuration, events, and actions to ensure a seamless user experience.
Updated 5 months ago
Next Step