Authentication
The Authentication section of the OpenWeb Android SDK provides mechanisms to manage user authentication, enabling seamless login experiences and secure access to the platform’s features.
This includes Single Sign-On (SSO), guest user management, and logout functionalities.
By integrating these APIs, publishers can:
- Implement their own custom login flow.
- Renew authentication tokens securely.
- Provide login prompts for guests.
- Manage user sessions effectively.
This section guides you through the key authentication capabilities, with examples and references to help you integrate them smoothly into your application.
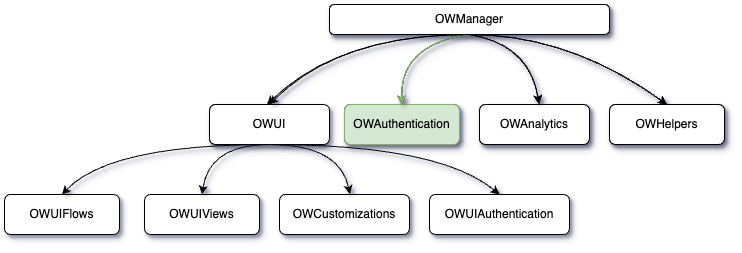
Authentication API Overview
Authentication in the OpenWeb Android SDK is managed through the OWAuthentication interface, which provides the following properties and methods:
- startSSO(callback: SpotCallback): Starts the SSO flow by generating a
codeA
. - completeSSO(codeB: String, callback: SpotCallback): Completes the SSO flow using
codeB
. - renewSSOAuthentication: A nullable lambda function to renew SSO authentication tokens.
- ssoWithJwt(jwt: String?, callback: SpotCallback): Allows JWT-based SSO authentication.
- shouldDisplayLoginPromptForGuests: A boolean flag to determine whether login prompts should be displayed for guest users in the full conversation view.
- getUserLoginStatus(callback: SpotCallback): Retrieves the current user login status (e.g., Guest or Registered).
- logout(callback: SpotVoidCallback): Logs the user out and clears session data.
SSO Authentication
Handshake Overview
- Initiate an OpenWeb SSO session. The OpenWeb SDK generates an SSO session ID (
codeA
). - Send the SSO session ID to your backend user management system.
- Validate that a user is logged into your system. You must ensure that the current user logged into your site will also be the current user in the OpenWeb user session.
- From your backend user management system, make a
GET /sso/v1/register-user
call to OpenWeb's backend. The API call must include the following information:- Your secret access token (
access_token
) - Session ID generated after initiating the SSO session (
code_a
) - Required user details from your backend user management system (
primary_key
,user_name
).
OpenWeb's backend logs in the user and returns a success response (codeB
).
- Your secret access token (
- Pass
codeB
to the OpenWeb SDK. Successfully completing this process results in logging in a user.
Implementation
The SSO flow involves generating codeA
, exchanging it for codeB
, and completing the authentication process with codeB
. This ensures secure and efficient authentication for registered users.
val authentication = OpenWeb.manager.authentication
// Start the SSO flow
authentication.startSSO(object : SpotCallback<StartSSOResponse> {
override fun onSuccess(response: StartSSOResponse) {
val codeA = response.codeA ?: return
// Use SSO session ID (`codeA`) to validate user is logged in.
// Complete the login process with OpenWeb.
// After successful login, execute the Complete SSO flow below.
val codeB = exchangeCodeAForCodeB(codeA)
// Complete the SSO flow
authentication.completeSSO(codeB, object : SpotCallback<String> {
override fun onSuccess(result: String) {
// SSO completed successfully
}
override fun onFailure(exception: SpotException) {
// Handle failure
}
})
}
override fun onFailure(exception: SpotException) {
// Handle failure
}
})
Renew SSO Authentication
Regardless of the SSO approach used, you must implement
OWSsoAuthenticator
.
Renewing SSO ensures uninterrupted user connectivity. It is triggered when the existing authenticator for a previously connected user becomes invalid. This process silently re-establishes the user's connection without requiring any direct action.
val authentication = OpenWeb.manager.authentication
authentication.renewSSOAuthentication = object : OWSsoAuthenticator {
override fun renew(userId: String, renewSSOListener: OWRenewSSOListener) {
// Follow the SSO authentication steps.
// After your flow completes successfully:
renewSSOListener.onComplete()
}
}
Third-party Provider SSO
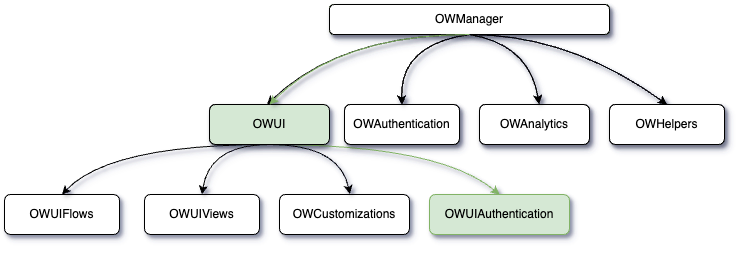
The ssoWithJwt
method allows authentication via third-party identity providers using a JWT (JSON Web Token). After authenticating the user with a third-party identity provider, pass the obtained JWT to the SDK to initiate the login.
val authentication = OpenWeb.manager.authentication
// Authenticate with a third-party JWT
authentication.ssoWithJwt(jwt, object : SpotCallback<SsoWithJwtResponse> {
override fun onSuccess(response: SsoWithJwtResponse) {
if (response.autoComplete) {
// SSO successful
}
}
override fun onFailure(exception: SpotException) {
// Handle failure
}
})
Custom Login Flow
You can define your own login flow by implementing the LoginDelegate
interface. This allows you to manage the user login process entirely within your application.
val authentication = OpenWeb.manager.ui.authentication
// Set a custom login delegate
authentication.setLoginDelegate(object : LoginDelegate {
override fun startLoginUIFlow(activityContext: Context) {
// Launch the custom login activity
val intent = Intent(activityContext, CustomLoginActivity::class.java)
activityContext.startActivity(intent)
}
})
Login Prompts for Guests
You can control whether a login prompt is displayed for guest users in the full conversation view using the shouldDisplayLoginPromptForGuests
property.
val authentication = OpenWeb.manager.authentication
// Enable login prompts for guest users
authentication.shouldDisplayLoginPromptForGuests = true
User Status
The getUserLoginStatus
method allows you to retrieve the current user status, which can be either Guest
or SSOLoggedIn
.
val authentication = OpenWeb.manager.authentication
// Get the current user status
authentication.getUserLoginStatus(object : SpotCallback<UserStatus> {
override fun onSuccess(status: UserStatus) {
when (status) {
is UserStatus.Guest -> {
// Handle guest user
}
is UserStatus.SSOLoggedIn -> {
val userId = status.userId
// Handle registered user
}
}
}
override fun onFailure(exception: SpotException) {
// Handle failure
}
})
Logout
The logout
method clears the current user session and logs the user out.
val authentication = OpenWeb.manager.authentication
// Log out the user
authentication.logout(object : SpotVoidCallback {
override fun onSuccess() {
// Successfully logged out
}
override fun onFailure(exception: SpotException) {
// Handle failure
}
})
Updated about 1 month ago