Conversation
Choose an approach to implement Conversation in your iOS app.
Conversation enables you to create a fluent conversation experience that fuels quality interactions with community and content and allows users to create valuable and engaging content:
- Convert casual visitors into loyal, registered readers
- Significantly increase the user time-on-site
- Provide lightweight, fully customizable features
- Increase pageviews and SEO rankings
- Enable automatic content moderation
- Encourage user engagement with your content and other users
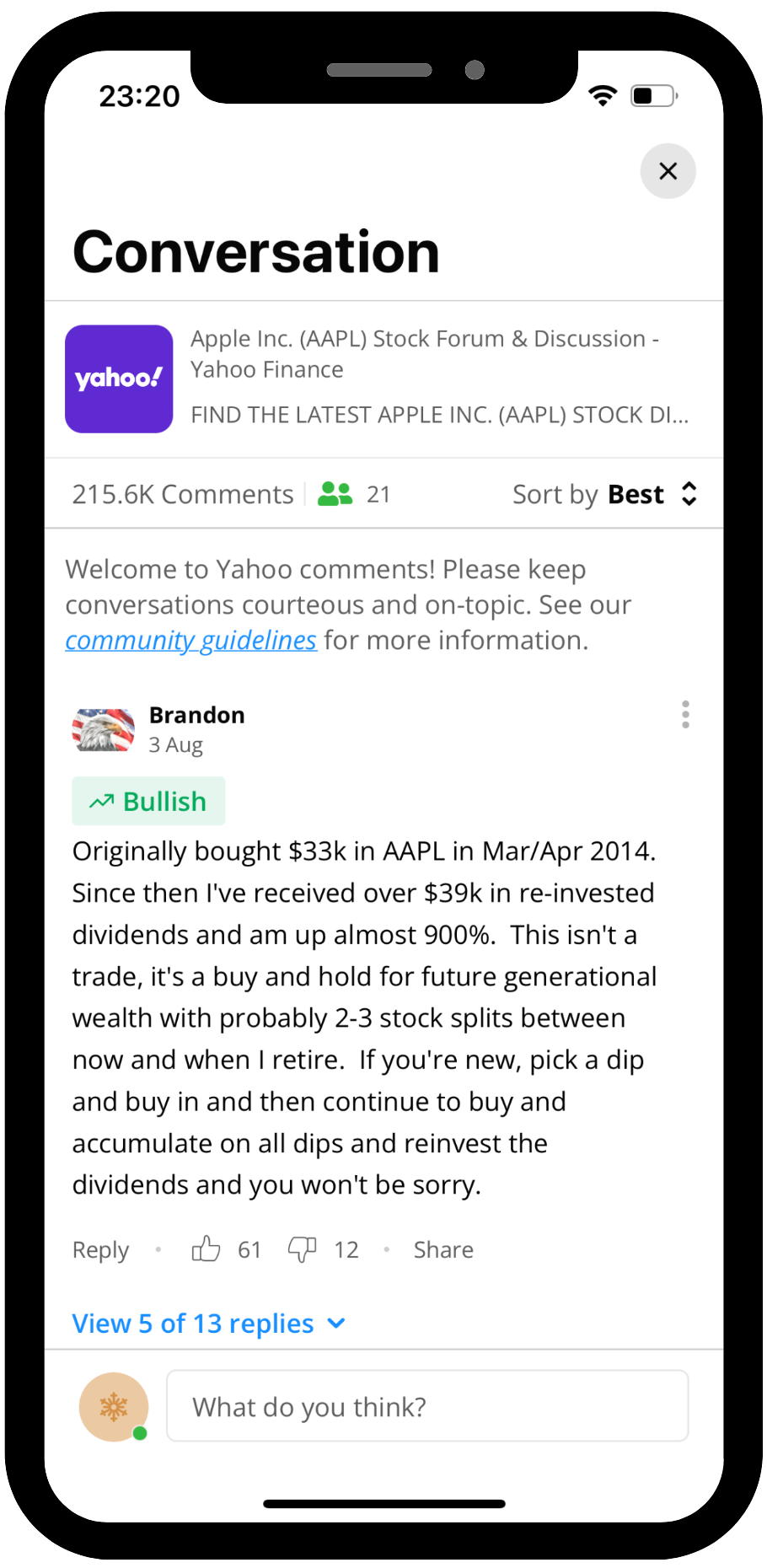
Full Conversation
Get started
- Define an article.
- Configure a flows or views implementation.
- Customize the UI.
- Configure user authentication.
- Add helpers to help streamline your development process and grants you extensive control over non-UI-related features:
- Configure analytics to get information about certain actions at the SDK side.
Updated 3 months ago