Customization options
Change default settings to enhance your brand
The OpenWeb Android SDK allows you to customize the appearance of different app features to create a seamless UI and branding experience for your users.
User experience
The ConversationOptions.Builder()
allows you to customize aspects of the conversation user experience, such as the theme and comment sort order.
Use the following steps to configure the appearance of the Pre-Conversation fragment and Conversation:
-
In your
Activity
orFragment
, useConversationOptions.Builder()
to create and define aConversationOptions
object namedoptions
.
Supported Configuration Settings:- Article Header Visibility
- Color Theme
- Comment Actions Customizations
- Current Article Details
- Custom Theme
- Language
- Locale
- Number of Displayed Comments
- PreConversation Style
- Read-only Status
- Sort Options Naming
- Sort Order
val themeMode = SpotImThemeMode.DARK val backgroundColor = Color.parseColor("#000000") val options = ConversationOptions.Builder() .addTheme(SpotImThemeParams(false, themeMode, backgroundColor)) .configureArticle( Article( "URL", "THUMBNAIL_URL", "TITLE", "SUBTITLE") ) .addSortType(SortType.SORT_NEWEST) .build()
-
Add the
options
argument to the Pre-Conversation fragment, Conversation, or create a comment screen in theActivity
.SpotIm.getPreConversationFragment(CONVERSATION_ID, options, object : SpotCallback<Fragment> { override fun onSuccess(fragment: Fragment) { // doSomething... } override fun onFailure(exception: SpotException) { // doSomething... } })
SpotIm.getConversationIntent(context, CONVERSATION_ID, options, object : SpotCallback<Intent> { override fun onSuccess(intent: Intent) { startActivity(intent) } override fun onFailure(exception: SpotException) { // Handle error here } })
SpotIm.getCreateCommentIntent(context, CONVERSATION_ID, options, object : SpotCallback<Intent> { override fun onSuccess(intent: Intent) { startActivity(intent) } override fun onFailure(exception: SpotException) { // Handle error here } })
-
(Optional) Monetize the Conversation.
Article Header Visibility
To hide the article header, set .setDisplayArticleHeader(false)
val options = ConversationOptions.Builder()
.setDisplayArticleHeader(false)
.build()
Color Theme
addTheme(SpotImThemeParams(isSupportSystemDarkMode, themeMode, backgroundColor))
sets up a SpotImThemeParams
class that sets the sorting background color and the Conversation theme.
val themeMode = SpotImThemeMode.DARK
val backgroundColor = parseColor("#000000")
val options = ConversationOptions.Builder()
.addTheme(SpotImThemeParams(false, themeMode, backgroundColor))
.build()
Argument | Description |
---|---|
isSupportSystemDarkMode Boolean | Defines if the Partner app supports system dark mode
This should be set to false to enable the themeMode argument to be defined by addTheme() .
|
themeMode | OpenWeb theme to be applied to the Pre-Conversation and Conversation
Possible values:
|
backgroundColor Int | Background color for the Pre-Conversation fragment and Conversation when the themeMode is SpotImThemeMode.DARK .
The value of this argument is ignored when the themeMode is SpotImThemeMode.LIGHT .
|
Comment Actions Customizations
setCommentActionsCustomizations()
defines the style and color comment actions buttons
val options = ConversationOptions.Builder()
.setCommentActionsCustomizations(
CommentActionsCustomizations(
commentActionsButtonsColor = CommentActionsButtonsColor.BRAND_COLOR,
commentActionsButtonsFont = CommentActionsButtonsFont.SEMI_BOLD
)
)
.build()
Argument | Description |
---|---|
CommentActionsButtonsColor CommentActionsButtonsColor | Color the comment actions button
Possible Values:
|
CommentActionsButtonsFont CommentActionsButtonsFont | Styling of the font
Possible Values:
|
Current Article Details
configureArticle(Article("URL", "THUMBNAIL_URL", "SUBTITLE"))
sets up an Article class that defines the current article.
Please contact your PSM to learn more about comment labels and set remote configurations related to comment sections or labels.
When custom labels are set up in the config, call configureArticleSection()
with a custom section name to enable or override the existing default section.
val options = ConversationOptions.Builder()
.configureArticleSection("SECTION_NAME")
.build()
In the following scenarios,
configureArticleSection
should not be called:
- If the default comment label section is set up in the config, comment labels will be enabled on all Conversations. There is no need to call
configureArticleSection
.- If the comment label config is not set up, calling
configureArticleSection
will have no effect.
Custom Theme
setCustomTheme()
defines the color to use for UI items in light (lightColor
) and dark (darkColor
) modes.
When customizing a
SpotImTheme
property, bothlightColor
anddarkColor
must be defined.
val options = ConversationOptions.Builder()
.setCustomTheme(
SpotImTheme(
voteUpSelectedColor = UIColor(lightColor = Color.BLACK, darkColor = Color.WHITE),
voteDownSelectedColor = UIColor(lightColor = Color.BLACK, darkColor = Color.WHITE),
voteUpUnselectedColor = UIColor(lightColor = Color.BLACK, darkColor = Color.WHITE),
voteDownUnselectedColor = UIColor(lightColor = Color.BLACK, darkColor = Color.WHITE)
)
)
.build()
Argument | Description |
---|---|
voteDownSelectedColor | Color of the vote down icon when selected |
voteDownUnselectedColor | Color of the vote down icon when not selected |
voteUpSelectedColor | Color of the vote up icon when selected |
voteUpUnselectedColor | Color of the vote up icon when not selected |
Language
setLanguageStrategy(OWLanguageStrategy)
defines how the SDK language is selected.
val options = ConversationOptions.Builder()
.setLanguageStrategy(OWLanguageStrategy.Device)
.build()
Argument | Description |
---|---|
OWLanguageStrategy OWLanguageStrategy | Options for language selection
Possible values:
|
* Regional variants of Portuguese are determined by the OWLocaleStrategy
. To allow users to choose their version of Portuguese, set OWLocaleStrategy
with .setLocaleStrategy(OWLocaleStrategy.Device)
. To force a specific version of Portuguese, set .setLocaleStrategy(OWLocaleStrategy.ServerConfig)
and contact your PSM to choose the specific version of Portuguese.
Locale
setLocaleStrategy(OWLocaleStrategy)
controls how the regional settings is selected.
val options = ConversationOptions.Builder()
.setLocaleStrategy(OWLocaleStrategy.Device)
.build()
Argument | Description |
---|---|
OWLocaleStrategy OWLocaleStrategy | Options for local selection
Possible values:
|
Number of Displayed Comments
addMaxCountOfPreConversationComments(counter)
limits the number of comments a Pre-Conversation fragment displays.
val options = ConversationOptions.Builder()
.addMaxCountOfPreConversationComments(4)
.build()
Argument | Description |
---|---|
counter Int | Integer between 0-16 that determines number of comments displayed:
|
PreConversation Style
setPreConversationStyle(OWPreConversationStyle)
sets the preConversation appearance.
val options = ConversationOptions.Builder()
.setPreConversationStyle(style)
.build()
Argument | Description |
---|---|
style OWPreConversationStyle | Initial appearance style
Possible values:
|
Read-only Status
setReadOnly(readOnlyMode)
controls whether additional comments can be added to a specific article.
val readOnlyMode = ReadOnlyMode.DEFAULT
val options = ConversationOptions.Builder()
.setReadOnly(readOnlyMode)
.build()
Argument | Description |
---|---|
readOnlyMode readOnlyMode | State of read-only mode
This attribute must be set to true to prohibit additional comments or replies from being posted.
Possible values:
|
Sort Options Naming
setCustomSortByOptionText(sortOption, R.string.customTitle)
establishes the custom name of a comment.
val options = ConversationOptions.Builder()
.setCustomSortByOptionText(SpotImSortOption.BEST, R.string.customTitle)
Argument | Description |
---|---|
sortOption SpotImSortOption | Type of sort order
Possible values:
|
r.string.customTitle | Custom text for comment name |
Sort Order
setInitialSort(sortOption)
sets up a SpotImSortOption
parameter that defines the initial sort order of comments.
val options = ConversationOptions.Builder()
.setInitialSort(SpotImSortOption.BEST)
.build()
Argument | Description |
---|---|
sortOption SpotImSortOption | Type of sort order
Possible values:
|
UI Elements
The CustomUIDelegate
allows you full access to customize UI components, such as the login prompt, Conversation footer, navigation title, and more. Customizing different components enables you to adjust elements as if the UI were your own.
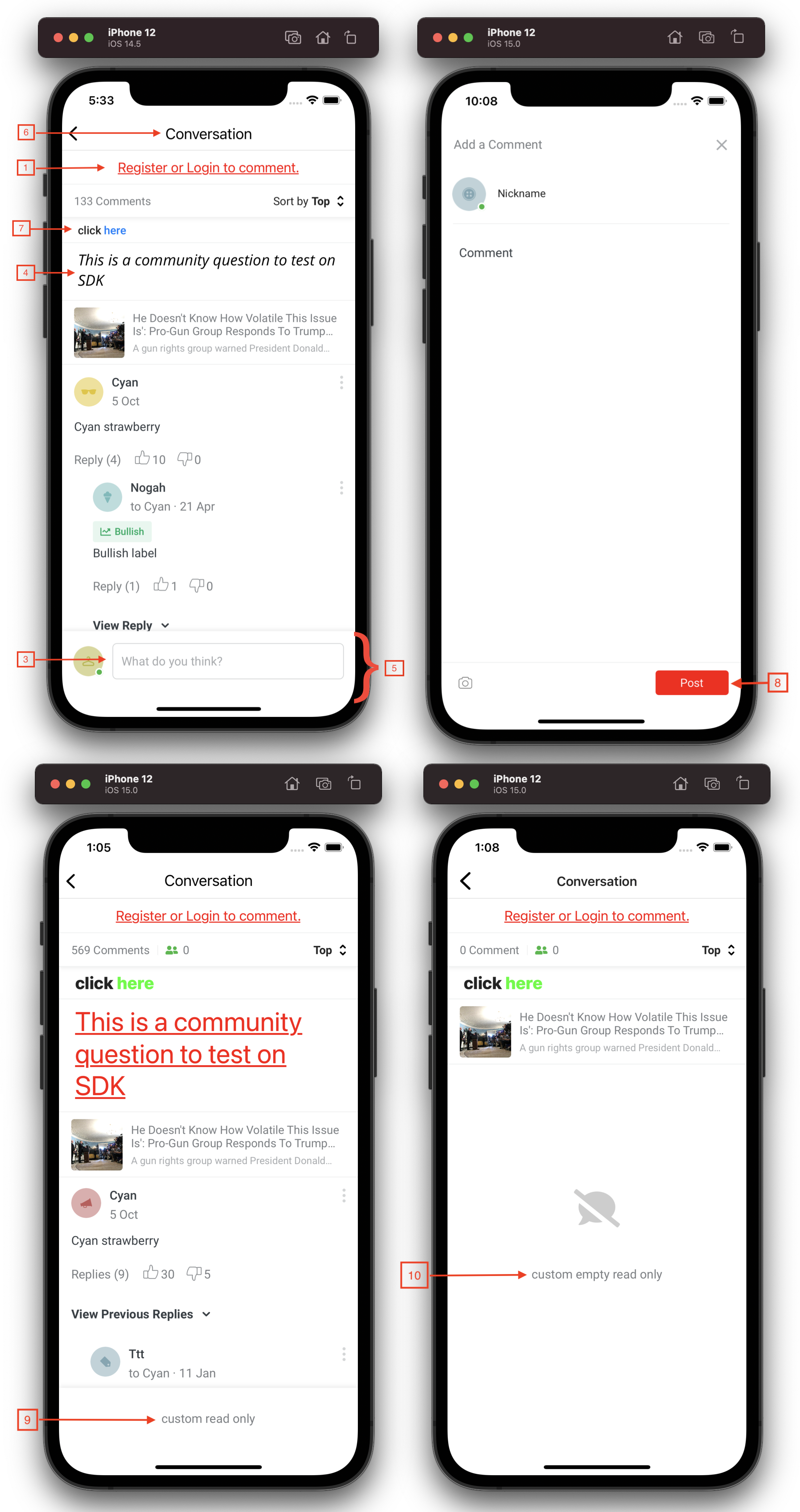
Use the following steps to configure the captions of different UI components:
- Set a
CustomUIDelegate
.
SpotIm.setCustomUIDelegate(object: CustomUIDelegate {
override fun customizeView(viewType: CustomizableViewType, view: View, isDarkModeEnabled: Boolean) {
when (viewType) {
CustomizableViewType.LOGIN_PROMPT_TEXT_VIEW -> {
val textView = view as? TextView
// set your own customization
}
// set more customizations to another CustomizableViewType
}
}
}
- Define and customize the
CustomizableViewType
.
CustomizableViewType Reference
# | CustomizableViewType option | CustomizableViewType script |
---|---|---|
1 | Login prompt TextView | LOGIN_PROMPT_TEXT_VIEW |
2 | Say control TextView in Pre-conversation | SAY_CONTROL_IN_PRE_CONVERSATION_TEXT_VIEW |
3 | Say control TextView in conversation | SAY_CONTROL_IN_CONVERSATION_TEXT_VIEW |
4 | Community question TextView | COMMUNITY_QUESTION_TEXT_VIEW |
5 | Conversation footer View | CONVERSATION_FOOTER_VIEW |
6 | Navigation title TextView | NAVIGATION_TITLE_TEXT_VIEW |
7 | Community guidelines TextView | COMMUNITY_GUIDELINES_TEXT_VIEW |
8 | Create Comment action button | COMMENT_CREATION_ACTION_BUTTON |
9 | Read-only label | READ_ONLY_TEXT_VIEW |
10 | Empty state read-only label | EMPTY_STATE_READ_ONLY_TEXT_VIEW |
11 | Pre-conversation header title TextView | PRE_CONVERSATION_HEADER_TEXT_VIEW |
12 | Pre-conversation header comments counter TextView | PRE_CONVERSATION_HEADER_COUNTER_TEXT_VIEW |
13 | Show more comments button | SHOW_COMMENTS_BUTTON |
Fonts
You can replace the OpenWeb Android SDK's default fonts with your own custom fonts. Using custom fonts tailors the viewer experience to your brand.
Use the following steps to add custom fonts to your app:
-
In the app module in Android Studio, add your font to the res/font directory.
In the following screenshot, three fonts have been added to the res/font directory:
- big_shoulder_bold.ttf
- big_shoulder_italic.ttf
- big_shoulder_regular.ttf
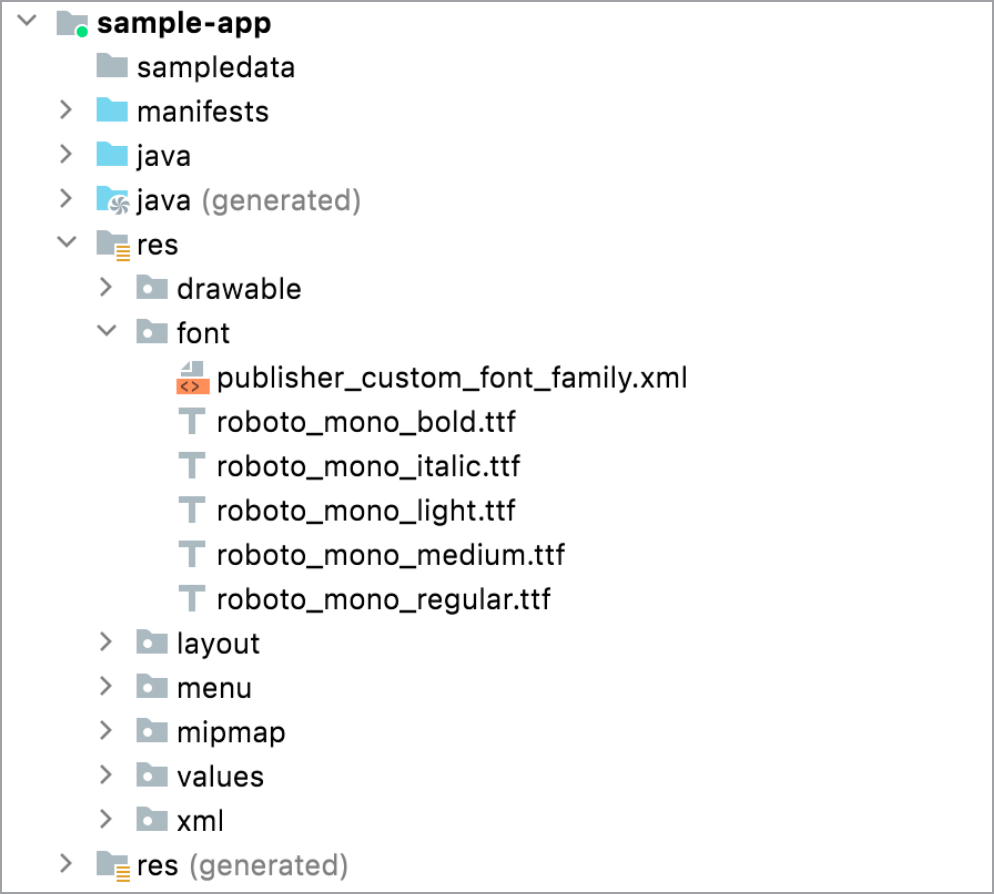
- Create publisher_custom_font_family.xml file with the following code.
<?xml version="1.0" encoding="utf-8"?>
<font-family xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto">
<!-- regular -->
<font
android:font="@font/big_shoulder_regular"
android:fontStyle="normal"
android:fontWeight="400"
app:font="@font/big_shoulder_regular"
app:fontStyle="normal"
app:fontWeight="400" />
<!-- italic -->
<font
android:font="@font/big_shoulder_italic"
android:fontStyle="normal"
android:fontWeight="400"
app:font="@font/big_shoulder_italic"
app:fontStyle="italic"
app:fontWeight="400" />
<!-- bold -->
<font
android:font="@font/big_shoulder_bold"
android:fontStyle="normal"
android:fontWeight="700"
app:font="@font/big_shoulder_bold"
app:fontStyle="normal"
app:fontWeight="700" />
</font-family>
- In res/values/styles.xml, add the following entries.
<style name="SpotIm.Theme.Dark.Language">
<item name="android:fontFamily">@font/publisher_custom_font_family</item>
<item name="fontFamily">@font/publisher_custom_font_family</item>
</style>
<style name="SpotIm.Theme.Light.Language">
<item name="android:fontFamily">@font/publisher_custom_font_family</item>
<item name="fontFamily">@font/publisher_custom_font_family</item>
</style>
Updated 9 months ago