Helpers
The OpenWeb SDK's OWHelpers
protocol helps streamline your development process.
This protocol grants you extensive control over non-UI-related features:
- Use Conversation Counters for real-time insights on replies and comments
- Customize logging strategies with the
OWLoggerConfiguration
- Define the UI language with various options through the
OWLanguageStrategy
- Adapt your app dates and numbers formats with options through the
OWLocaleStrategy
public protocol OWHelpers {
func conversationCounters(forPostIds postIds: [OWPostId],
completion: @escaping OWConversationCountersCompletion)
func conversationCounters(forPostIds postIds: [OWPostId]) async throws
-> [OWPostId: OWConversationCounter]
var additionalConfigurations: [OWAdditionalConfiguration] { get set }
var loggerConfiguration: OWLoggerConfiguration { get }
var languageStrategy: OWLanguageStrategy { get set }
var localeStrategy: OWLocaleStrategy { get set }
var orientationEnforcement: OWOrientationEnforcement { get set }
}
The following sections explain how to configure and define these settings.
OWHelpers
Conversation Counters
To show your users the engagement levels of specific Conversations, you can display the number of user comments that have been posted to all requested conversations.
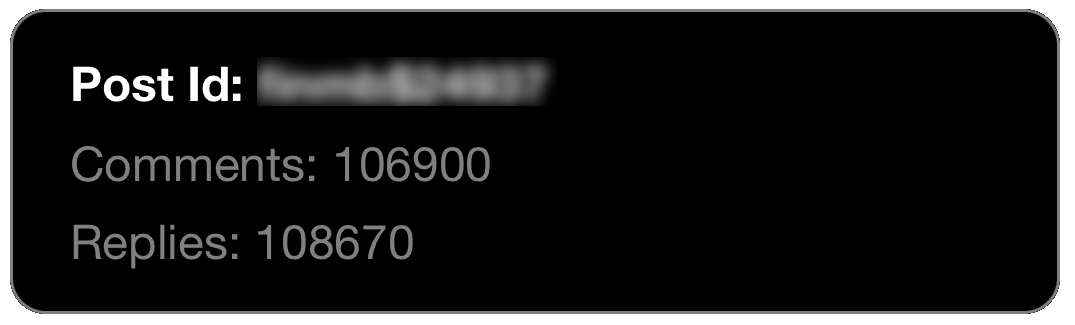
By referencing the post IDs you can add the user comment counts for those Conversations.
let manager: OWManagerProtocol = OpenWeb.manager
let helpers: OWHelpers = manager.helpers
// Conversation counters
let conversationCountersClosure: OWConversationCountersCompletion = { result in
switch result {
case .success(let counters):
// All good
let postIds: [String] = Array(counters.keys)
for postId in postIds {
let counter: OWConversationCounter = counters[postId]!
let repliesNum = counter.replies
let commentsNum = counter.comments
// Update your UI / Do the work you need
}
case .failure(let error):
// Handle the error / Show appropriate user UI
break
}
}
helpers.conversationCounters(forPostIds: ["firstId", "secondId"],
completion: conversationCountersClosure)
let manager: OWManagerProtocol = OpenWeb.manager
let helpers: OWHelpers = manager.helpers
// Conversation counters
do {
let counters = try await helper.conversationCounters(forPostIds: postIds)
let postIds: [String] = Array(counters.keys)
for postId in postIds {
let counter: OWConversationCounter = counters[postId]!
let repliesNum = counter.replies
let commentsNum = counter.comments
// Update your UI / Do the work you need
}
} catch {
// Handle the error / Show appropriate user UI
}
Logging
When you configure the logger, OpenWeb disregards any pending logs. OpenWeb will not create files for these logs.
We strongly recommend configuring the logger after the initialization method. This ensures that files will be created for any pending logs.
Without impacting app performance, the iOS SDK logging system can help you troubleshoot your implementation of the iOS SDK. No logs are sent to OpenWeb's backend servers.
The iOS log files offer the following benefits:
- Viewing logs in the console.
- Saving logs locally in the application's Document directory.
- Generating logs that can be submitted with a support ticket when issues are hard to reproduce.
- Help OpenWeb improve the stability of future SDK builds.
The logging system has minimal performance impact:
- Log files are created in the
OpenWebSDKLoggerFileCreationQueue
queue with a.background
priority. - All other logging functions are performed in the
OpenWebSDKLoggerQueue
background queue with a.utility
priority.
let manager: OWManagerProtocol = OpenWeb.manager
let helpers: OWHelpers = manager.helpers
let loggerConfig: OWLoggerConfiguration = helpers.loggerConfiguration
loggerConfig.level = .verbose
loggerConfig.methods = [.nsLog, .file(maxFilesNumber: 20)]
Setting | Description |
---|---|
level string | Determines the amount and type of information logged by the iOS SDK
Possible Values:
Default: .verbose
|
methods array | Defines the methods by which the logs are stored or displayed
While the following options can all be used together, we recommend using one of the following combinations:
Possible Values:
Default: logMethods: [.nsLog, .file(maxFilesNumber: 20)]
|
Expand the following sections to learn more information about NSLog, OSLog, and the files.
NSLog
The following screenshots show the Xcode debugger console and device logs when using the NSLog framework.

Xcode debugger console
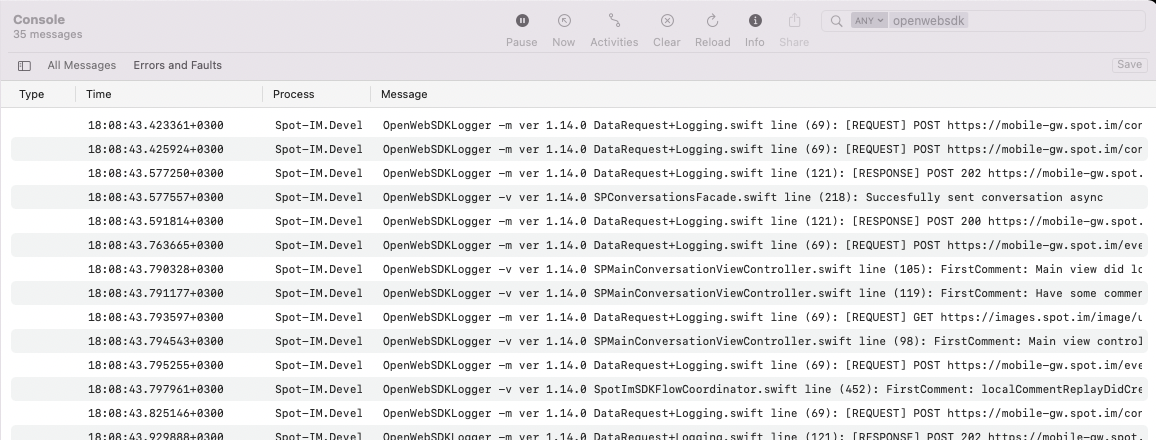
Device logs
OSLog
The following screenshots show the Xcode debugger console and device logs when using Apple's preferred OSLog framework.

Xcode debugger console
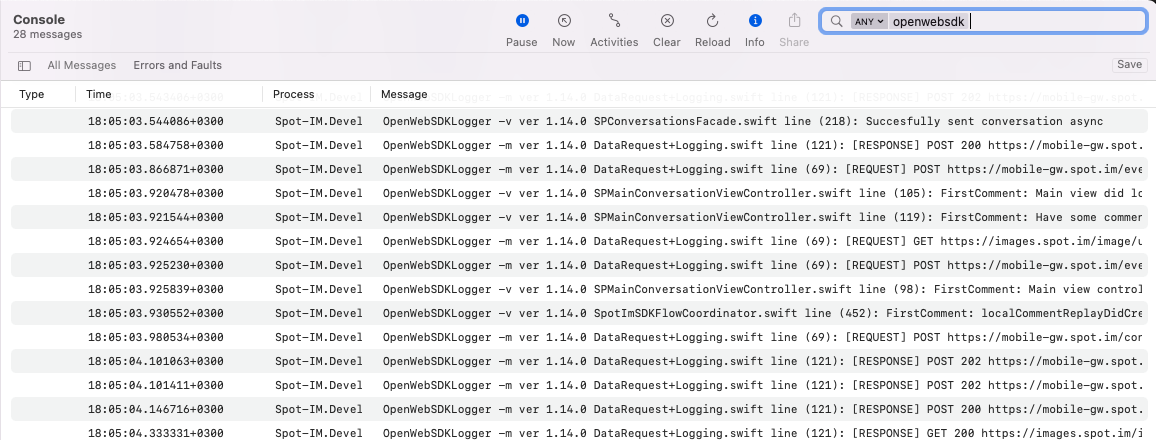
Device logs
Files
This option defines the maximum number of log files that will be created and stored in your application's Documents directory. After exceeding this number, the logger will remove the oldest log file when creating a new log file.
The logger will create a file in either of the following conditions:
- There are 100 items* to log.
* An item is each message logged by OpenWeb, such as a simple line or full information about a network request. The
level
affects the data within each item and if the item is logged. Based on testing,level: .verbose
will not generate log files that exceed 1.5MB. - The application has moved to the background.
The following screenshots are examples from inside a simulator directory.
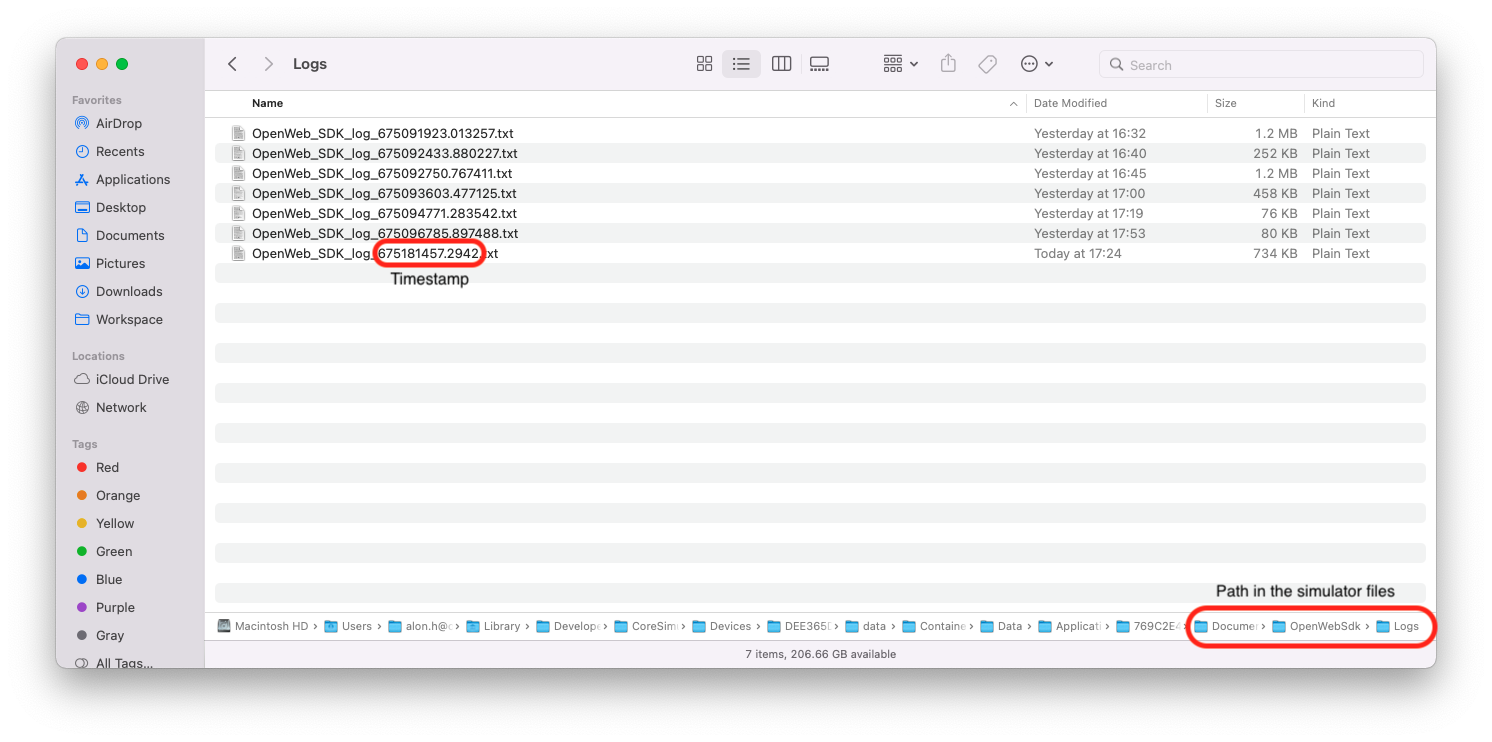
Simulator directory
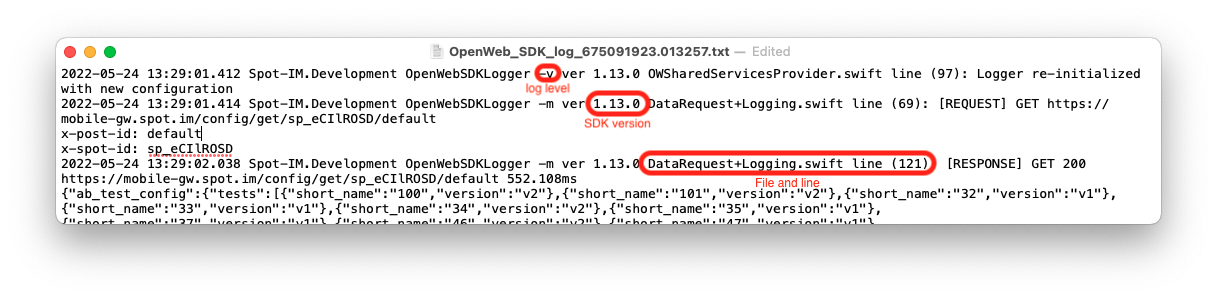
Log file
Language
With multiple-language support, OWLanguageStrategy
allows you to set the language of the user interface for your audience.
let manager: OWManagerProtocol = OpenWeb.manager
let helpers: OWHelpers = manager.helpers
helpers.languageStrategy = OWLanguageStrategy
Setting | Description |
---|---|
OWLanguageStrategy OWLanguageStrategy | Defines the language of the user interface
See: OWLanguageStrategy |
Locale
Ensuring that dates and numbers appear in familiar formats based on user preferences or device settings enhances the user experience. The OWLocaleStrategy
enables you to set these preferences for your users.
let manager: OWManagerProtocol = OpenWeb.manager
let helpers: OWHelpers = manager.helpers
helpers.localeStrategy = OWLocaleStrategy
Setting | Description |
---|---|
OWLocaleStrategy OWLocaleStrategy | Defines the locale which will be used for dates and numbers formats
See: OWLocaleStrategy |
Orientation
OWOrientationEnforcement
allows you to set the orientation of the user interface for your audience.
let manager: OWManagerProtocol = OpenWeb.manager
let helpers: OWHelpers = manager.helpers
helpers.orientationEnforcement: OWOrientationEnforcement
Setting | Description |
---|---|
OWOrientationEnforcement OWOrientationEnforcement | Defines orientation of the UI
See: OWOrientationEnforcement |
Additional Configurations
For apps requiring tailored, publisher-specific setups, OpenWeb offers the flexibility of custom configurations. When applicable, your OpenWeb PSM will furnish the required values for the OWAdditionConfiguration.
let manager: OWManagerProtocol = OpenWeb.manager
let helpers: OWHelpers = manager.helpers
helpers.additionalConfigurations: [OWAdditionalConfiguration] = []
Setting | Description |
---|---|
OWAdditionalConfiguration OWAdditionalConfiguration | Defines custom, publisher-specific configurations
If needed for your app, your OpenWeb PSM will provide the necessary values for the OWAdditionConfiguration
|
Enumerations
OWConversationCountersCompletion
typealias OWConversationCountersCompletion = (
Result<[OWPostId: OWConversationCounter], OWError>
) -> Void
OWConversationCounter
public struct OWConversationCounter {
public let commentsNumber: Int
public let repliesNumber: Int
}
Setting | Description |
---|---|
commentsNumber Integer | Number of comments |
repliesNumber Integer | Number of replies |
OWLanguageStrategy
Setting | Description |
---|---|
.useDevice | (Default) Uses the language defined on the user device |
.use(language: OWSupportedLanguage) | Permits defining the language of the user interface |
.useServerConfig | Uses the language defined in the Admin Panel |
OWLocaleStrategy
Setting | Description |
---|---|
.useDevice | (Default) Uses the locale defined on the user device |
.use(locale: Locale) | Permits defining the locale |
.useServerConfig | Uses the local defined in the Admin Panel, dictated by the language in the Admin Panel |
OWOrientation
Setting | Description |
---|---|
OWOrientation string | Options for setting the UI orientation
Possible Values:
|
OWOrientationEnforcement
Setting | Description |
---|---|
OWOrientationEnforcement string | Options for setting the UI orientation
Possible Values:
|
OWSupportedLanguage
Setting | Description |
---|---|
OWSupportedLanguage string | Options for setting the UI language
Possible Values:
|
Updated about 1 month ago