SDK Structure
Understand the organization of the OpenWeb iOS SDK.
The OpenWeb iOS SDK is comprised of layers that conform to various protocols.
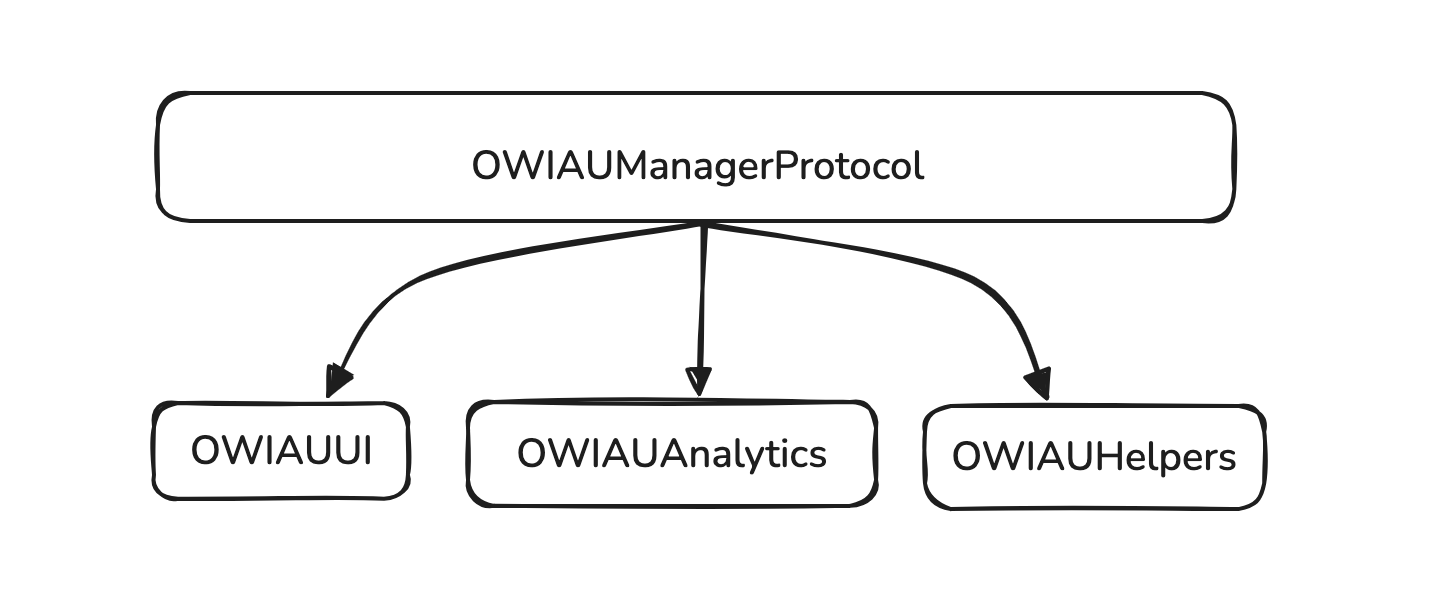
The primary protocol is the OWIAUManagerProtocol
. OpenWebIAU.manager
is the gateway for developers to interface with the SDK. This manager is a singleton class that conforms to the OWIAUManagerProtocol
.
public protocol OWIAUManagerProtocol {
var spotId: OWIAUSpotId { get set }
var ui: OWIAUUI { get }
var analytics: OWIAUAnalytics { get }
var settings: OWIAUSettingsProtocol { get set }
var helpers: OWIAUHelpers { get }
}
By leveraging the manager, you can engage and interact seamlessly with various layers of the SDK.
Subsequent sections of the documentation dive deeper into each of these layers.
Request Tracking Authorization
To display an ad, you must first present the tracking authorization dialog to the user:
import AppTrackingTransparency
import AdSupport
...
// AppDelegate::applicationDidBecomeActive, or:
// UIViewController::viewDidLoad (provided it happens after applicationDidBecomeActive)
ATTrackingManager.requestTrackingAuthorization { status in
// Tracking authorization completed. Start loading ads here.
}
Initialize the SDK
Your spotId
is provided by your PSM. This ID is crucial as it's the primary identifier for interactions. The manager assists in setting this spotId
.
In addition to the spotId
, you also need to configure your Store URL using the OWIAUSettingsBuilder
.
Important
It's imperative to set the
spotId
and your Store URL via the manager before invoking any other part of the API.
import OpenWebIAUSDK
var manager = OpenWebIAU.manager
manager.spotId = // Some Spot id
var settingsBuilder = OWIAUSettingsBuilder()
let exampleStoreURL: String = // your store url
settingsBuilder.storeURL(exampleStoreURL)
manager.settings = settingsBuilder.build()
Updated 5 months ago