Flows and Views
The OWUIFlows
and OWUIViews
interfaces provide two approaches to integrating the Conversation module and other UI elements into your app: Flows and Views.
Each approach enables you to incorporate elements such as PreConversation, Conversation, and Comment Creation experiences, tailored to your specific requirements.
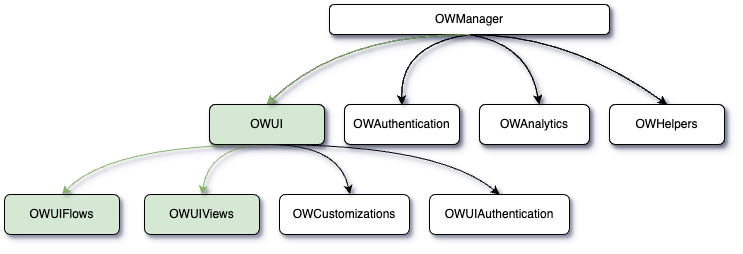
Choosing Between Flows and Views
Flows
Flows manage complete user journeys automatically. Use this approach when:
- You need an experience where navigation is handled for you.
- You want the SDK to manage transitions and user flow efficiently.
Views
Views provide flexibility to control and customize individual screens. Use this approach when:
- You need embedded components that integrate seamlessly into your app.
- You want to manage navigation and user interactions manually.
- You need granular control over how the UI elements are displayed within your app.
Comparing Flows and Views
The table below provides a comparison between flows and views.
Feature | Flows | Views |
---|---|---|
Use Case | Managed navigation and transitions | Embedded components |
Navigation | Handled by the SDK | Managed by the publisher |
Customization | Limited | Full control |
Integration Effort | Minimal | Moderate to advanced |
Flows
The OWUIFlows
interface manages full-screen transitions and interactions, making it ideal for publishers seeking a straightforward integration with minimal development effort.
Available Methods: Intents
Method | Description |
---|---|
getConversation | Generates an Intent for the full-page Conversation experience. |
getCommentThread | Generates an Intent for a comment thread, starting from the root comment. |
getCreateComment | Generates an Intent for the Comment Creation flow. |
Example:
OpenWeb.manager.ui.flows.intents.getConversation(
postId = "POST_ID",
articleSettings = articleSettings,
additionalSettings = OWAdditionalSettings(),
flowActionsCallback = object : OWFlowActionsCallbacks {
override fun callback(type: OWFlowActionCallbackType, source: OWFlowSourceType, postId: OWPostId) {
// Handle flow-specific actions
}
},
callback = object : SpotCallback<Intent> {
override fun onSuccess(intent: Intent) {
startActivity(intent)
}
override fun onFailure(exception: SpotException) {
// Handle errors
}
}
)
Available Methods: Fragments
Method | Description |
---|---|
getPreConversation | Retrieves a Fragment for a preview of comments at the end of an article. |
getConversation | Retrieves a Fragment for the full-page Conversation experience. |
Example:
OpenWeb.manager.ui.flows.fragments.getConversation(
postId = "POST_ID",
articleSettings = articleSettings,
additionalSettings = OWAdditionalSettings(),
flowActionsCallback = object : OWFlowActionsCallbacks {
override fun callback(type: OWFlowActionCallbackType, source: OWFlowSourceType, postId: OWPostId) {
// Handle flow-specific actions
}
},
callback = object : SpotCallback<Fragment> {
override fun onSuccess(fragment: Fragment) {
supportFragmentManager.beginTransaction()
.replace(R.id.fragment_container, fragment)
.commit()
}
override fun onFailure(exception: SpotException) {
// Handle errors
}
}
)
Views
The OWUIViews
interface provides granular control over individual UI components. Use this approach to embed elements such as PreConversation, Conversation, or Comment Creation views directly into your app.
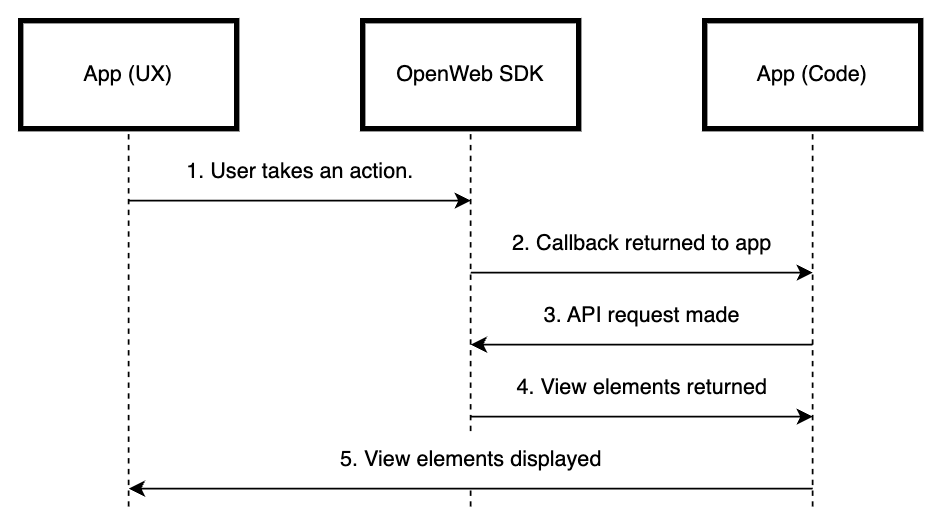
In this workflow, the following occurs:
- A user takes an action, such as tapping a button.
- The user's action triggers OpenWeb's callbacks API to return a callback, signifying an event occurred.
- As the developer, you use this event to make another API call to OpenWeb.
- OpenWeb's API responds with the specific view element related to the API call.
- As the developer, you manage the presentation of the views or elements returned by OpenWeb. You can decide whether these views or elements are displayed or hidden from the user.
Available Methods
Method | Description |
---|---|
getPreConversation | A preview of comments to encourage engagement. |
getConversation | The main view for the Conversation. |
getCommentCreation | A view for entering a comment. |
getCommentThread | Displays a comment thread starting from a specific comment. |
getReportReasons | A screen listing possible report options. |
Example:
OpenWeb.manager.ui.views.getConversation(
postId = "POST_ID",
articleSettings = articleSettings,
conversationSettings = OWConversationSettings(),
viewActionsCallbacks = object : OWViewActionsCallbacks {
override fun callback(type: OWViewActionCallbackType, source: OWViewSourceType, postId: OWPostId) {
// Handle user actions within the view
}
},
callback = object : SpotCallback<Fragment> {
override fun onSuccess(fragment: Fragment) {
supportFragmentManager.beginTransaction()
.replace(R.id.fragment_container, fragment)
.commit()
}
override fun onFailure(exception: SpotException) {
// Handle errors
}
}
)
Property Descriptions
Property (Type) | Description |
---|---|
postId (OWPostId) | Unique identifier for the article associated with the flow or view. The ideal postId has the following characteristics:- Aligns with the URL slug ( an-article-title ) or article ID (14325 )- Is less than 50 characters, ideally 15 characters - Uses a combination of letters, numbers, dashes (-), or hyphens (_) - Doesn't include special characters, excluding the following regex: [^\w\s-:.$\~] |
articleSettings (OWArticleSettings) | Configuration details for the associated article. Includes the article's URL, title, and additional settings. See: OWArticleSettings. |
preConversationSettings (OWPreConversationSettings) | Configuration for the PreConversation component, including styles and the number of comments displayed. See: OWPreConversationSettings. |
conversationSettings (OWConversationSettings) | Settings related to the Conversation component, such as layout style, engagement options, and spacing. See: OWConversationSettings. |
commentThreadSettings (OWCommentThreadSettings) | Configuration for the Comment Thread component, defining behaviors for nested discussions. See: OWCommentThreadSettings. |
commentCreationSettings (OWCommentCreationSettings) | Configuration options for the Comment Creation component, including layout options like light mode or floating keyboard. See: OWCommentCreationSettings. |
commentCreationType (OWCommentCreationType) | Specifies the type of comment operation (e.g., comment, edit, or reply) that is performed within the Comment Creation component. |
commentId (String) | Unique identifier for a comment. Used to target a specific comment in flows such as commentThread or reportReason. |
reportReasonsInfo (ReportReasonsInfo) | Provides information and options for the Report Reasons screen, including a list of reportable reasons for moderation. |
additionalSettings (OWAdditionalSettings) | Defines the settings for each component in a flow or view. Possible Values: - commentCreationSettings - conversationSettings - preConversationSettings |
flowActionsCallback (OWFlowActionsCallbacks?) | Handles flow-specific actions, such as navigation triggers or dismissing flows. |
viewActionsCallbacks (OWViewActionsCallbacks?) | Handles user-specific actions within views (e.g., button presses or navigation events). |
callback (SpotCallback) | A callback function that handles success or failure for a flow or view operation. |
Enumerations and Structs
OWAdditionalSettings
Setting | Description |
---|---|
preConversationSettings | Sets the initial preConversation flow settings. Possible Values: - Regular (default) - Compact - CtaButtonOnly - CtaWithSummary |
conversationSettings | Sets the initial Conversation flow settings. Possible Values: - Regular (default) - Compact - Custom (with guidelines and spacing) |
commentCreationSettings | Sets the initial comment creation flow settings. Possible Values: - Regular (default) - Floating - Light |
OWCommunityGuidelinesStyle
Setting | Description |
---|---|
None | Disables community guidelines display. |
Compact | Displays guidelines in a compact format. |
Regular | Displays guidelines in the standard format. |
OWCommunityQuestionsStyle
Setting | Description |
---|---|
Compact | Displays questions in a compact format. |
Regular | Displays questions in the standard format. |
OWConversationSpacing
Setting | Description |
---|---|
Regular | Standard spacing between elements. |
Compact | Reduced spacing for a tighter layout. |
Custom | User-defined spacing for fine-grained control. |
Updated 3 months ago