Analytics
The OWIAUAnalytics
protocol in the OpenWeb iOS IAU SDK allows you to track analytics events and set custom business intelligence (BI) data, enabling integration with your internal analytics systems.
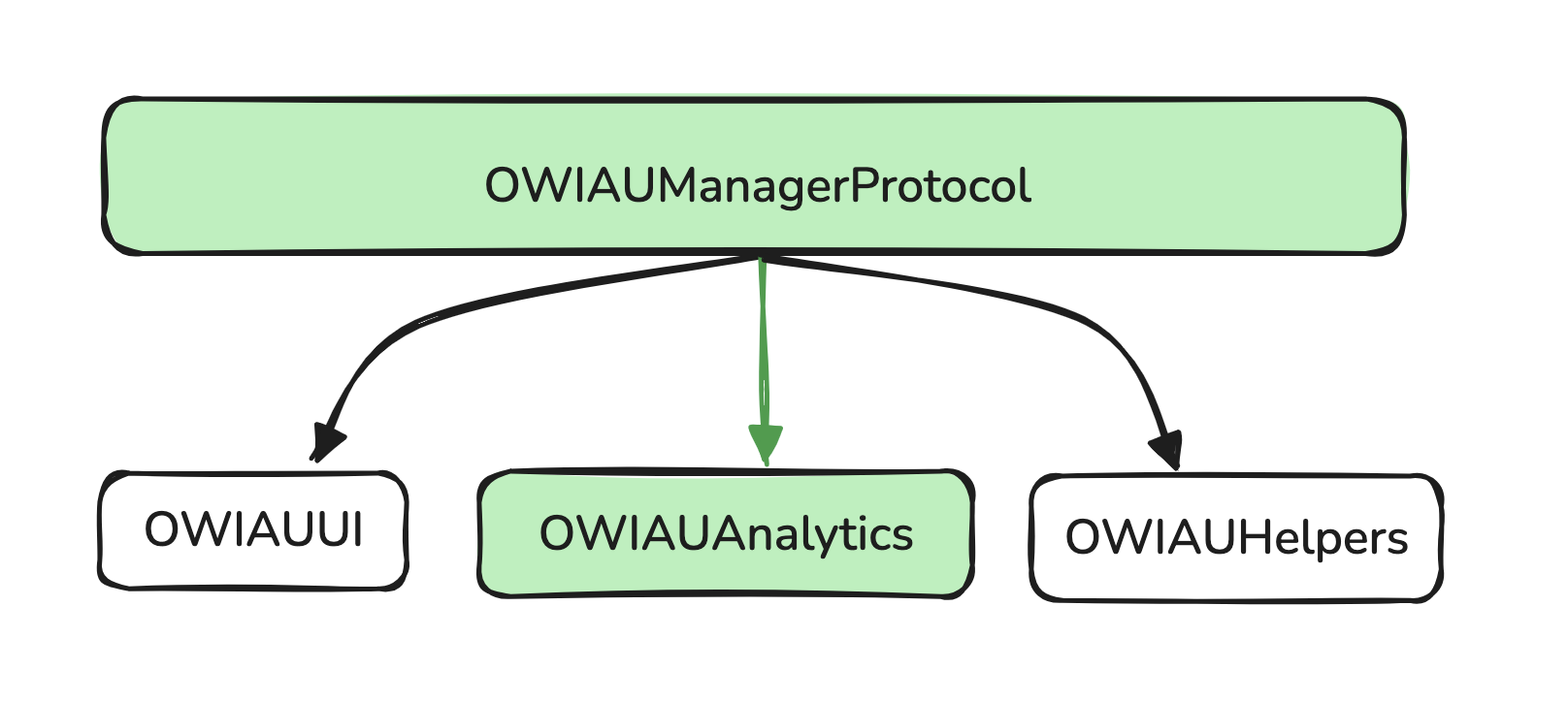
Protocol Definition
Defines the structure for managing analytics events and custom BI data.
public protocol OWIAUAnalytics {
var customBIData: OWIAUCustomBIData { get set }
func addBICallback(_ callback: @escaping OWIAUAnalyticEventCallback)
}
Tracking Analytics Events
You can use the OWIAUAnalytics
protocol to assign BI data and register callbacks to handle and track specific analytics events.
import OpenWebIAUSDK
// Analytics
var analytics: OWIAUAnalytics = OpenWeb.manager.analytics
// Set custom BI
let data: OWIAUCustomBIData = ...
analytics.customBIData = data
// Set callback for the events
let analyticClosure: OWIAUAnalyticEventCallback = { event, additionalInfo, postId in
switch event {
case .generalSdkLoaded:
// Send your own BI/analytics here
case // some other event type
case .default:
break
}
}
analytics.addBICallback(analyticClosure) // Allow multiple callbacks
Enumerations and Structs
These enumerations and structures define the possible analytics events and the associated callback behavior.
OWIAUBIAnalyticEvent
Lists the events you can track, enabling detailed analytics integration.
Possible event values include:
.generalSdkLoaded
.generalCampaignConfigsFetched
.generalEngineMonetizationLoad
.generalMonetizedPageView
.generalMonetizedPageViewDelayed
.dfpEngineWillInitialize
.dfpSlotRequested
.dfpEmpty
.dfpImpressionViewable
.dfpSlotResponseReceived
.dfpSlotOnLoad
.dfpSlotRenderEnded
.dfpInitialLoadDisabled
.aniviewEngineWillInitialize
.aniviewAdClickThroughVideo
.aniviewEngineDone
.aniviewEngineClosed
.aniviewAdInventoryVideo
.aniviewContentClicked
.aniviewContentCompleted
.aniviewContentImpression
.aniviewEngineError
.aniviewEngineImpression
.aniviewEngineInitializeError
.aniviewEngineInitialized
OWBIAnalyticEventCallback
Defines the format of callbacks for handling analytics events.
public typealias OWIAUAnalyticEventCallback = (
event: OWIAUBIAnalyticEvent,
additionalInfo: OWIAUAnalyticAdditionalInfoProtocol,
postId: OWIAUPostId?
) -> Void
OWIAUAnalyticAdditionalInfoProtocol
- Provides additional information for analytics events, customized by the publisher.PostID
- A unique identifier for an article, used to associate events with specific pages.OWIAUCustomBIData
- Specifies the structure for custom BI data used in analytics:
typealias OWCustomBIData = [String: Codable]
Updated 6 months ago
Next Step