Debugging and Logs
Troubleshoot your implementation of the OpenWeb iOS SDK
The following sections explain how to use the SDK's logging system for debugging and troubleshoot common errors that can occur during implementation.
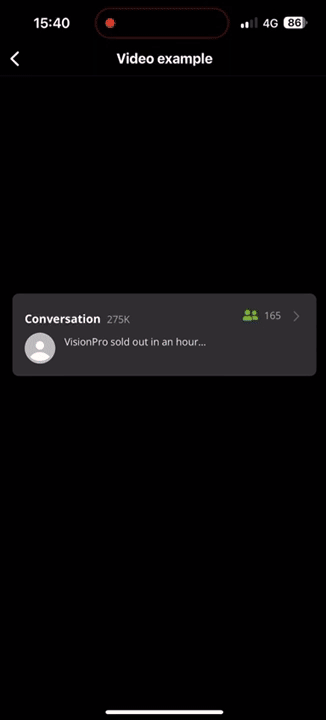
Logging
Without impacting app performance, the iOS SDK logging system can help you troubleshoot your implementation of the iOS SDK. No logs are sent to OpenWeb's backend servers.
The iOS log files offer the following benefits:
- Viewing logs in the console.
- Saving logs locally in the application's
Document
directory. - Generating logs that can be submitted with a support ticket when issues are hard to reproduce.
- Help OpenWeb improve the stability of future SDK builds.
The logging system has minimal performance impact:
- Log files are created in the
OpenWebSDKLoggerFileCreationQueue
queue with a.background
priority. - All other logging functions are performed in the
OpenWebSDKLoggerQueue
background queue with a.utility
priority.
Configure logging settings
When you configure the logger, OpenWeb disregards any pending logs. OpenWeb will not create files for these logs.
We strongly recommend configuring the logger after the initialization method. This ensures that files will be created for any pending logs.
By default, OpenWeb initializes the logger with the following settings:
logLevel: .verbose
logMethods: [.nsLog, .file(maxFilesNumber: 20)]
To configure these default logging settings, you can call the following method.
SpotIm.configureLogger(logLevel: SPLogLevel, logMethods: [SPLogMethod])
SPLogLevel
Option | Description | Debug Symbol |
---|---|---|
none |
No logged items | -n |
error |
Logged items:
|
-e |
medium |
Logged items:
|
-m |
verbose |
Logged items:
|
-v |
SPLogMethod
While the following options can all be used together, we recommend using one of the following combinations:
[.nsLog, file(maxFilesNumber: Int)]
[.osLog, file(maxFilesNumber: Int)]
Option | Description |
---|---|
nsLog |
Apple NSLog |
osLog |
Preferred Apple OSLog framework |
file(maxFilesNumber: Int) |
Defines the maximum number of log files that will be created and stored in your application's Documents directorymaxFilesNumber : Maximum number log files that are stored locallyThe maxFilesNumber must be a number within the following range: 1-200. If the value passed is outside of this range, the default value of 20 will be used. |
NSLog
The following screenshots show the Xcode debugger console and device logs when using the NSLog framework.

Xcode debugger console
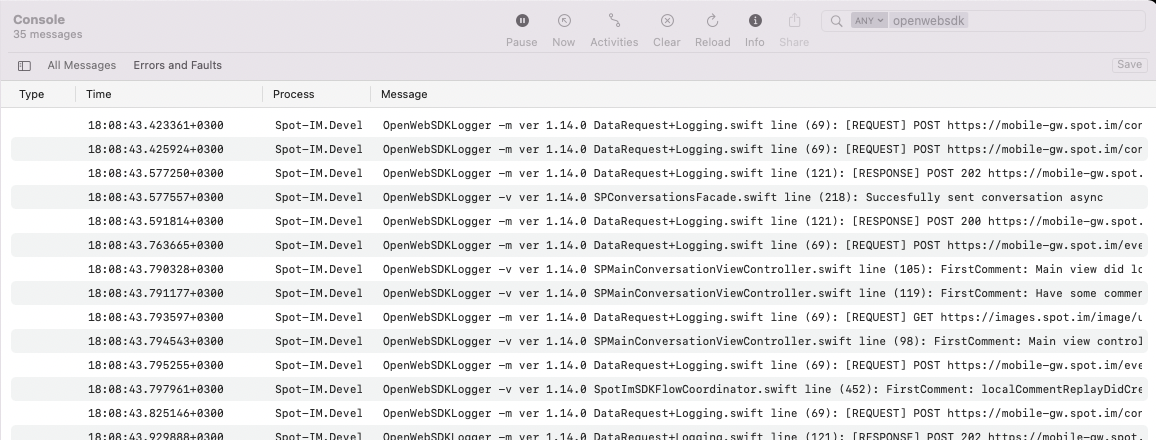
Device logs
OSLog
The following screenshots show the Xcode debugger console and device logs when using Apple's preferred OSLog framework.

Xcode debugger console
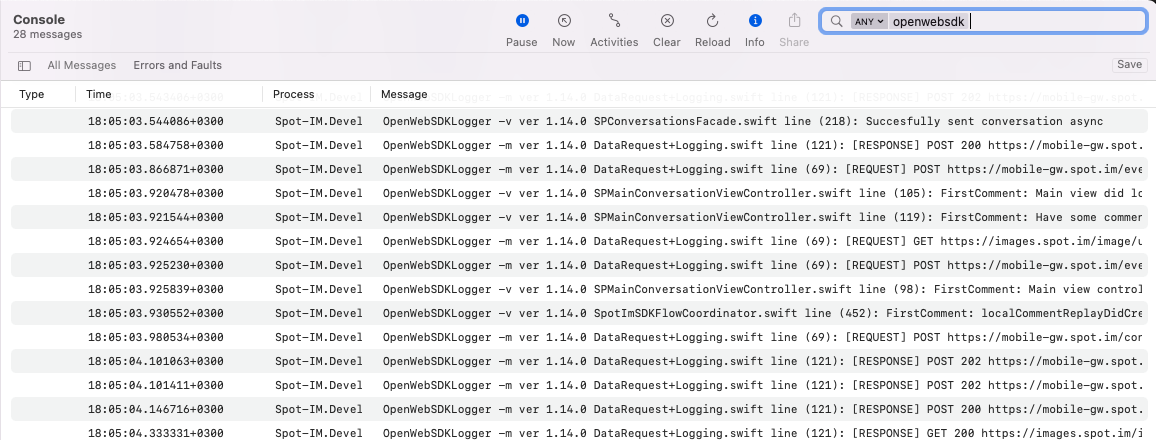
Device logs
Files
This option defines the maximum number of log files that will be created and stored in your application's Documents directory. After exceeding this number, the logger will remove the oldest log file when creating a new log file.
The logger will create a file in either of the following conditions:
- There are 100 items* to log.
* An item is each message logged by OpenWeb, such as a simple line or full information about a network request. The
SPLogLevel
affects the data within each item and if the item is logged. Based on testing,logLevel: .verbose
will not generate log files that exceed 1.5MB. - The application has moved to the background.
The following screenshots are examples from inside a simulator directory.
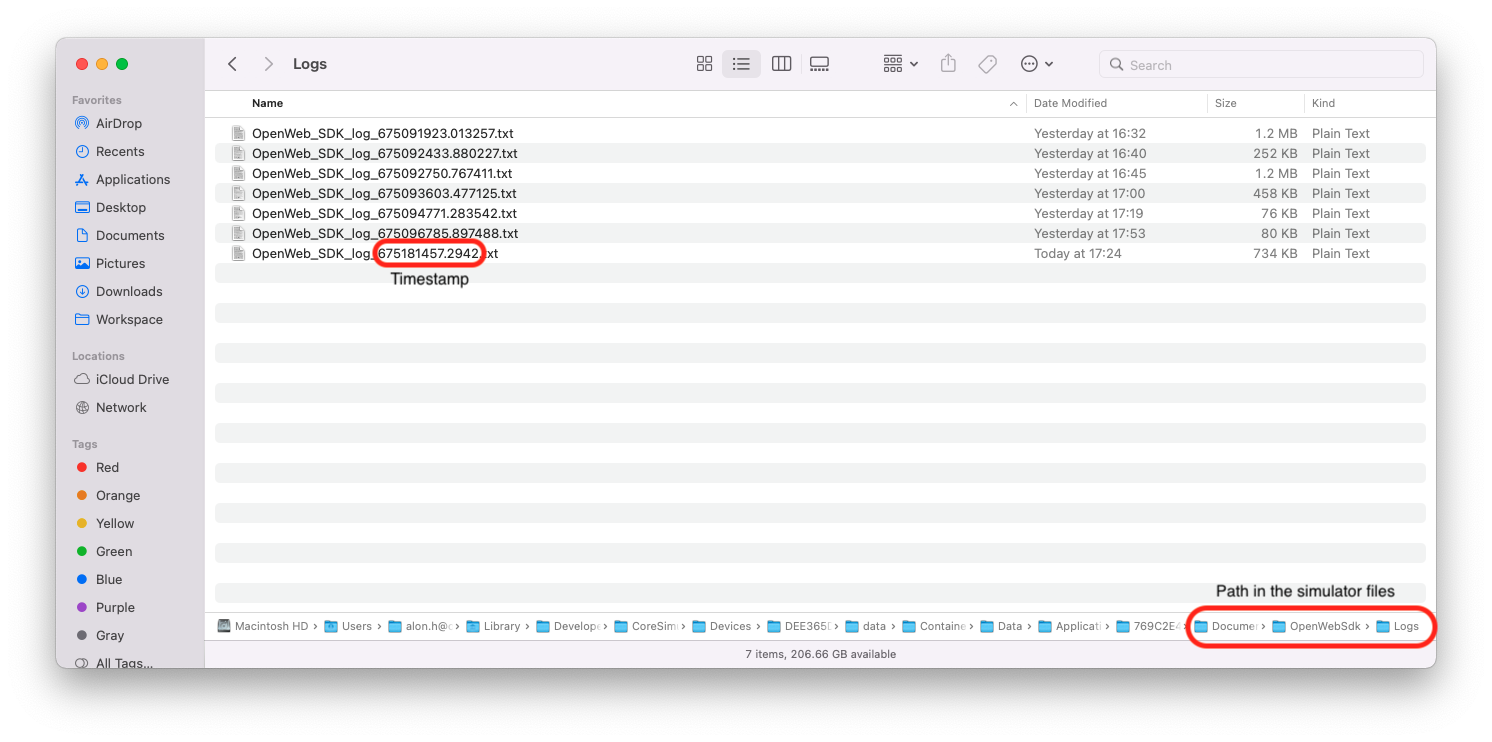
Simulator directory
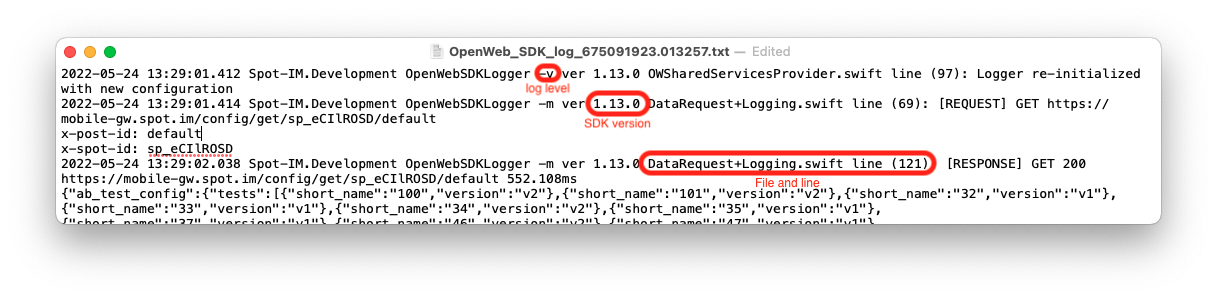
Log file
Disable logging
Log files can be an essential debugging tool. At minimum, we recommend enabling log files in your debug environment.
If you need to disable logging, you can call the following method.
SpotIm.configureLogger(logLevel: .none , logMethods: [])
Troubleshooting
The examples below provide solutions to common errors when implementing the iOS SDK.
CocoaPods installation fails with error
ERROR:
[!] Unable to find a specification for `SpotIMCore...
SOLUTION:
Run pod repo update
in Terminal to refresh your local spec repos before installation.
Pod search fails with error
ERROR:
[!] Unable to find a pod with name, author, summary, or description matching `SpotIMCore`
SOLUTION:
The CocoaPods search index has known bugs. Simply proceed with the usual installation.
Xcode build fails with error
ERROR:
Failed to build module 'SpotImCore'; this SDK is not supported by the compiler (the SDK is built with 'Apple Swift version 5.5 (swiftlang-1300.0.31.1 clang-1300.0.29.1)', while this compiler is 'Apple Swift version 5.5.2 (swiftlang-1300.0.47.5 clang-1300.0.29.30)'). Please select a toolchain which matches the SDK.
SOLUTION:
In your Podfile, ensure that you are using Frameworks.
// For static linkage
use_frameworks! :linkage => :static
// Or for dynamic linkage
use_frameworks!
If using post-install, exclude SpotIMCore and its related dependencies from the IPHONEOS_DEPLOYMENT_TARGET configuration. See this example post-install:
post_install do |installer|
update_os_licences
installer.generated_projects.each do |project|
if project.project_name != "SpotIMCore" && project.project_name != "Alamofire" && project.project_name != "PromiseKit" && project.project_name != "RxCocoa" && project.project_name != "RxSwift" && project.project_name != "RxRelay"
project.build_configurations.each do |config|
config.build_settings['CLANG_WARN_QUOTED_INCLUDE_IN_FRAMEWORK_HEADER'] = 'YES'
config.build_settings['IPHONEOS_DEPLOYMENT_TARGET'] = '12.0'
end
project.targets.each do |target|
target.deployment_target = '12.0'
target.build_configurations.each do |config|
config.build_settings['IPHONEOS_DEPLOYMENT_TARGET'] = '12.0'
end
end
end
end
end
Updated about 1 year ago